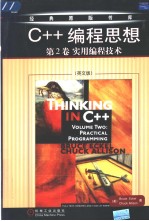
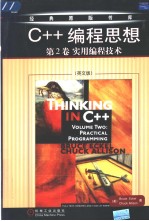
Thinking in C++ Volume 2:Practical ProgrammingPDF电子书下载
- 电子书积分:22 积分如何计算积分?
- 作 者:[美]埃克尔(Eckel
- 出 版 社:机械工业出版社
- 出版年份:2004
- ISBN:
- 页数:806 页
Introduction 1
Goals 1
Chapters 2
Exercises 5
Exercise solutions 5
Source code 5
Compilers 7
Language standards 9
Seminars, CD-ROMs & consulting 9
Errors 10
About the cover 10
Acknowledgements 10
Ⅰ:Building Stable Systems 13
1:Exception Handling 15
Traditional error handling 16
Throwing an exception 18
Catching an exception 20
The try block 20
Exception handlers 20
Termination and resumption 22
Exception matching 23
Catching any exception 25
Rethrowing an exception 26
Uncaught exceptions 26
Cleaning up 28
Resource management 30
Making everything an object 32
auto_ptr 35
Function-level try blocks 36
Standard exceptions 38
Exception specifications 40
Better exception specifications? 45
Exception specifications and inheritance 46
When not to use exception specifications 47
Exception safety 48
Programming with exceptions 52
When to avoid exceptions 52
Typical uses of exceptions 54
Overhead 58
Summary 60
Exercises 61
2:Defensive Programming 63
Assertions 66
A simple unit test framework 70
Automated testing 71
The TestSuite Framework 75
Test suites 79
The test framework code 81
Debugging techniques 87
Trace macros 87
Trace file 88
Finding memory leaks 90
Summary 96
Exercises 97
Ⅱ:The Standard C++ Library 101
3:Strings in Depth 103
What's in a string? 104
Creating and initializing C+ + strings 106
Operating on strings 109
Appending, inserting, and concatenating strings 110
Replacing string characters 112
Concatenation using nonmember overloaded operators 117
Searching in strings 117
Finding in reverse 123
Finding first/last of a set of characters 124
Removing characters from strings 126
Comparing strings 129
Strings and character traits 134
A string application 140
Summary 145
Exercises 146
4:Iostreams 151
Why iostreams? 151
Iostreams to the rescue 156
Inserters and extractors 156
Common usage 161
Line-oriented input 164
Handling stream errors 165
File iostreams 168
A File-Processing Example 169
Open modes 171
Iostream buffering 173
Seeking in iostreams 175
String iostreams 179
Input string streams 180
Output string streams 182
Output stream formatting 186
Format flags 186
Format fields 188
Width, fill, and precision 190
An exhaustive example 191
Manipulators 194
Manipulators with arguments 196
Creating manipulators 199
Effectors 201
Iostream examples 203
Maintaining class library source code 204
Detecting compiler errors 208
A simple data logger 211
Internationalization 216
Wide Streams 216
Locales 218
Summary 221
Exercises 222
5:Templates in Depth 227
Template parameters 227
Non-type template parameters 228
Default template arguments 230
Template template parameters 232
The typename keyword 238
Using the template keyword as a hint 240
Member Templates 242
Function template issues 245
Type deduction of function template arguments 245
Function template overloading 249
Taking the address of a generated function template 251
Applying a function to an STL sequence 255
Partial ordering of function templates 259
Template specialization 260
Explicit specialization 261
Partial Specialization 263
A practical example 265
Preventing template code bloat 268
Name lookup issues 273
Names in templates 273
Templates and friends 279
Template programming idioms 285
Traits 285
Policies 291
The curiously recurring template pattern 294
Template metaprogramming 297
Compile-time programming 298
Expression templates 308
Template compilation models 315
The inclusion model 315
Explicit instantiation 316
The separation model 319
Summary 320
Exercises 321
6:Generic Algorithms 325
A first look 325
Predicates 329
Stream iterators 331
Algorithm complexity 333
Function objects 335
Classification of function objects 336
Automatic creation of function objects 338
Adaptable function objects 341
More function object examples 343
Function pointer adaptors 351
Writing your own function object adaptors 358
A catalog of STL algorithms 362
Support tools for example creation 365
Filling and generating 368
Counting 370
Manipulating sequences 372
Searching and replacing 377
Comparing ranges 385
Removing elements 389
Sorting and operations on sorted ranges 393
Heap operations 403
Applying an operation to each element in a range 405
Numeric algorithms 413
General utilities 417
Creating your own STL-style algorithms 419
Summary 420
Exercises 421
7: Generic Containers 429
Containers and iterators 429
STL reference documentation 431
A first look 432
Containers of strings 438
STL containers 440
A plethora of iterators 442
Iterators in reversible containers 445
Iterator categories 446
Predefined iterators 448
The basic sequences: vector, list, deque 454
Basic sequence operations 454
vector 457
deque 465
Converting between sequences 467
Checked random-access 470
list 471
Swapping sequences 477
set 479
A completely reusable tokenizer 482
stack 487
queue 491
Priority queues 496
Holding bits 506
bitset<n> 507
vector<bool> 511
Associative containers 513
Generators and fillers for associative containers 518
The magic of maps 521
Multimaps and duplicate keys 523
Multisets 527
Combining STL containers 530
Cleaning up containers of pointers 534
Creating your own containers 536
STL extensions 538
Non-STL containers 540
Summary 546
Exercises 546
Ⅲ: Special Topics 549
8:Runtime Type Identification 551
Runtime casts 551
The typeid operator 557
Casting to intermediate levels 560
void pointers 561
Using RTTI with templates 562
Multiple inheritance 563
Sensible uses for RTTI 564
A trash recycler 565
Mechanism and overhead of RTTI 570
Summary 570
Exercises 571
9: Multiple Inheritance 573
Perspective 573
Interface inheritance 575
Implementation inheritance 579
Duplicate subobjects 585
Virtual base classes 589
Name lookup issues 599
Avoiding MI 603
Extending an interface 603
Summary 608
Exercises 609
10: Design Patterns 613
The pattern concept 613
Prefer composition to inheritance 615
Classifying patterns 615
Features, idioms,patterns 616
Simplifying Idioms 617
Messenger 617
Collecting Parameter 618
Singleton 619
Variations on Singleton 621
Command: choosing the operation 626
Decoupling event handling with Command 628
Object decoupling 631
Proxy: fronting for another object 632
State: changing object behavior 634
Adapter 636
Template Method 639
Strategy: choosing the algorithm at runtime 640
Chain of Responsibility: trying a sequence of strategies 642
Factories: encapsulating object creation 645
Polymorphic factories 647
Abstract factories 651
Virtual constructors 654
Builder: creating complex objects 660
Observer 667
The "inner class" idiom 671
The observer example 674
Multiple dispatching 679
Multiple dispatching with Visitor 683
Summary 687
Exercises 688
11: Concurrency 691
Motivation 692
Concurrency in C++ 694
Installing ZThreads 695
Defining Tasks 696
Using Threads 698
Creating responsive user interfaces 700
Simplifying with Executors 702
Yielding 706
Sleeping 707
Priority 709
Sharing limited resources 711
Ensuring the existence of objects 711
Improperly accessing resources 715
Controlling access 719
Simplified coding with Guards 721
Thread local storage 724
Terminating tasks 727
Preventing iostream collision 727
The ornamental garden 728
Terminating when blocked 733
Interruption 735
Cooperation between threads 741
Wait and signal 742
Producer-consumer relationships 747
Solving threading problems with queues 750
Broadcast 757
Deadlock 764
Summary 770
Exercises 773
A:Recommended Reading 777
General C++ 777
Bruce's books 777
Chuck's books 779
In-depth C++ 779
Design Patterns 781
B: Etc 783
Index 791
- 《SQL与关系数据库理论》(美)戴特(C.J.Date) 2019
- 《魔法销售台词》(美)埃尔默·惠勒著 2019
- 《看漫画学钢琴 技巧 3》高宁译;(日)川崎美雪 2019
- 《优势谈判 15周年经典版》(美)罗杰·道森 2018
- 《社会学与人类生活 社会问题解析 第11版》(美)James M. Henslin(詹姆斯·M. 汉斯林) 2019
- 《海明威书信集:1917-1961 下》(美)海明威(Ernest Hemingway)著;潘小松译 2019
- 《迁徙 默温自选诗集 上》(美)W.S.默温著;伽禾译 2020
- 《上帝的孤独者 下 托马斯·沃尔夫短篇小说集》(美)托马斯·沃尔夫著;刘积源译 2017
- 《巴黎永远没个完》(美)海明威著 2017
- 《剑桥国际英语写作教程 段落写作》(美)吉尔·辛格尔顿(Jill Shingleton)编著 2019
- 《高等教育双机械基础课程系列教材 高等学校教材 机械设计课程设计手册 第5版》吴宗泽,罗圣国,高志,李威 2018
- 《中国十大出版家》王震,贺越明著 1991
- 《近代民营出版机构的英语函授教育 以“商务、中华、开明”函授学校为个案 1915年-1946年版》丁伟 2017
- 《新工业时代 世界级工业家张毓强和他的“新石头记”》秦朔 2019
- 《智能制造高技能人才培养规划丛书 ABB工业机器人虚拟仿真教程》(中国)工控帮教研组 2019
- 《AutoCAD机械设计实例精解 2019中文版》北京兆迪科技有限公司编著 2019
- 《陶瓷工业节能减排技术丛书 陶瓷工业节能减排与污染综合治理》罗民华著 2017
- 《全国职业院校工业机器人技术专业规划教材 工业机器人现场编程》(中国)项万明 2019
- 《国之重器出版工程 云化虚拟现实技术与应用》熊华平 2019
- 《新闻出版博物馆 总第33期》新闻出版博物馆 2018