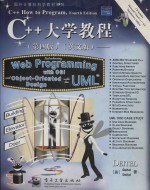
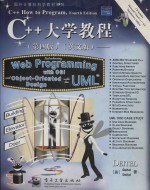
C++ how to programPDF电子书下载
- 电子书积分:32 积分如何计算积分?
- 作 者:Fourth Edition = C++大学教程(第四版)(英文版)
- 出 版 社:Publishing House of Electronics Industry
- 出版年份:2005
- ISBN:7121017598
- 页数:1342 页
1 Introduction to Computers and C++ Programming 1
1.1 Introduction 2
1.2 What is a Computer? 4
1.3 Computer Organization 5
1.4 Evolution of Operating Systems 6
1.5 Personal Computing,Distributed Computing and Client/Server Computing 7
1.6 Machine Languages,Assembly Languages,and High-level Languages 7
1.7 History of C and C++ 8
1.8 C++ Standard Library 10
1.9 Java 11
1.10 Visual Basic,Visual C++ and C 11
1.11 Other High-level Languages 13
1.12 Structured Programming 13
1.13 The Key Software Trend:Object Technology 14
1.14 Basics of a Typical C++ Environment 15
1.15 Hardware Trends 17
1.16 History of the Internet 18
1.17 History of the World Wide Web 19
1.18 World Wide Web Consortium (W3C) 20
1.19 General Notes About C++ and This Book 20
1.20 Introduction to C++ Programming 21
1.21 A Simple Program:Printing a Line of Text 21
1.22 Another Simple Program:Adding Two Integers 26
1.23 Memory Concepts 30
1.24 Arithmetic 31
1.25 Decision Making:Equality and Relational Operators 34
1.26 Thinking About Objects:Introduction to Object Technology and the Unified Modeling Language 40
1.27 Tour of the Book 44
2 Control Structures 70
2.1 Introduction 71
2.2 Algorithms 72
2.3 Pseudocode 72
2.4 Control Structures 73
2.5 if Selection Structure 76
2.6 if/else Selection Structure 77
2.7 while Repetition Structure 81
2.8 Formulating Algorithms:Case Study 1 (Counter-Controlled Repetition) 83
2.9 Formulating Algorithms with Top-Down,Stepwise Refinement:Case Study 2 (Sentinel-Controlled Repetition) 86
2.10 Formulating Algorithms with Top-Down,Stepwise Refinement:Case Study 3 (Nested Control Structures) 94
2.11 Assignment Operators 98
2.12 Increment and Decrement Operators 99
2.13 Essentials of Counter-Controlled Repetition 102
2.14 for Repetition Structure 104
2.15 Examples Using the for Structure 109
2.16 switch Multiple-Selection Structure 113
2.17 do/while Repetition Structure 120
2.18 break and continue Statements 122
2.19 Logical Operators 124
2.20 Confusing Equality (==) and Assignment (=) Operators 127
2.21 Structured-Programming Summary 128
2.22 [Optional Case Study] Thinking About Objects:Identifying a System’s Classes from a Problem Statement 133
3 Functions 169
3.1 Introduction 170
3.2 Program Components in C++ 170
3.3 Math Library Functions 171
3.4 Functions 173
3.5 Function Definitions 174
3.6 Function Prototypes 178
3.7 Header Files 180
3.8 Random Number Generation 182
3.9 Example:Game of Chance and Introducing enum 188
3.10 Storage Classes 192
3.11 Scope Rules 195
3.12 Recursion 198
3.13 Example Using Recursion:Fibonacci Series 202
3.14 Recursion vs.Iteration 206
3.15 Functions with Empty Parameter Lists 208
3.16 Inline Functions 209
3.17 References and Reference Parameters 211
3.18 Default Arguments 215
3.19 Unary Scope Resolution Operator 217
3.20 Function Overloading 219
3.21 Function Templates 222
3.22 [Optional Case Study] Thinking About Objects:Identifying a Class’s Attributes 225
4 Arrays 252
4.1 Introduction 253
4.2 Arrays 253
4.3 Declaring Arrays 255
4.4 Examples Using Arrays 256
4.5 Passing Arrays to Functions 272
4.6 Sorting Arrays 276
4.7 Case Study:Computing Mean,Median and Mode Using Arrays 278
4.8 Searching Arrays:Linear Search and Binary Search 283
4.9 Multiple-Subscripted Arrays 289
4.10 [Optional Case Study] Thinking About Objects:Identifying the Operations of a Class 296
5 Pointers and Strings 319
5.1 Introduction 320
5.2 Pointer Variable Declarations and Initialization 320
5.3 Pointer Operators 322
5.4 Calling Functions by Reference 325
5.5 Using const with Pointers 329
5.6 Bubble Sort Using Pass-by-Reference 336
5.7 Pointer Expressions and Pointer Arithmetic 341
5.8 Relationship Between Pointers and Arrays 344
5.9 Arrays of Pointers 349
5.10 Case Study:Card Shuffling and Dealing Simulation 350
5.11 Function Pointers 355
5.12 Introduction to Character and String Processing 360
5.12.1 Fundamentals of Characters and Strings 360
5.12.2 String Manipulation Functions of the String-Handling Library 362
5.13 [Optional Case Study] Thinking About Objects:Collaborations Among Objects 370
6 Classes and Data Abstraction 404
6.1 Introduction 405
6.2 Structure Definitions 406
6.3 Accessing Structure Members 407
6.4 Implementing User-Defined Type Time with a C-like struct 408
6.5 Implementing Abstract Data Type Time with a class 411
6.6 Class Scope and Accessing Class Members 418
6.7 Separating Interface from Implementation 420
6.8 Controlling Access to Members 424
6.9 Access Functions and Utility Functions 426
6.10 Initializing Class Objects:Constructors 430
6.11 Using Default Arguments with Constructors 430
6.12 Destructors 435
6.13 When Constructors and Destructors Are Called 435
6.14 Using Set and Get Functions 439
6.15 Subtle Trap:Returning a Reference to a private Data Member 445
6.16 Default Memberwise Assignment 448
6.17 Software Reusability 450
6.18 [Optional Case Study] Thinking About Objects:Starting to Program the Classes for the Elevator Simulator 451
7 Classes:Part Ⅱ 468
7.1 Introduction 469
7.2 const (Constant) Objects and const Member Functions 469
7.3 Composition:Objects as Members of Classes 478
7.4 friend Functions and friend Classes 485
7.5 Using the this Pointer 489
7.6 Dynamic Memory Management with Operators new and delete 495
7.7 static Class Members 497
7.8 Data Abstraction and Information Hiding 502
7.8.1 Example:Array Abstract Data Type 504
7.8.2 Example:String Abstract Data Type 504
7.8.3 Example:Queue Abstract Data Type 505
7.9 Container Classes and Iterators 505
7.10 Proxy Classes 506
7.11 [Optional Case Study] Thinking About Objects:Programming the Classes for the Elevator Simulator 509
8 Operator Overloading; String and Array Objects 546
8.1 Introduction 547
8.2 Fundamentals of Operator Overloading 548
8.3 Restrictions on Operator Overloading 549
8.4 Operator Functions as Class Members vs.as friend Functions 550
8.5 Overloading Stream-Insertion and Stream-Extraction Operators 552
8.6 Overloading Unary Operators 555
8.7 Overloading Binary Operators 555
8.8 Case Study:Array Class 556
8.9 Converting between Types 568
8.10 Case Study:string Class 569
8.11 Overloading ++ and -- 581
8.12 Case Study:A Date Class 582
8.13 Standard Library Classes string and vector 588
9 Object-Oriented Programming:Inheritance 609
9.1 Introduction 610
9.2 Base Classes and Derived Classes 611
9.3 protected Members 614
9.4 Relationship between Base Classes and Derived Classes 614
9.5 Case Study:Three-Level Inheritance Hierarchy 637
9.6 Constructors and Destructors in Derived Classes 642
9.7 “Uses A” and “Knows A” Relationships 648
9.8 public,protected and private Inheritance 648
9.9 Software Engineering with Inheritance 649
9.10 [Optional Case Study] Thinking About Objects:Incorporating Inheritance into the Elevator Simulation 650
10 Object-Oriented Programming:Polymorphism 662
10.1 Introduction 663
10.2 Relationships Among Objects in an Inheritance Hierarchy 664
10.2.1 Invoking Base-Class Functions from Derived-Class Objects 665
10.2.2 Aiming Derived-Class Pointers at Base-Class Objects 670
10.2.3 Derived-Class Member-Function Calls via Base-Class Pointers 672
10.2.4 Virtual Functions 673
10.3 Polymorphism Examples 679
10.4 Type Fields and switch Structures 680
10.5 Abstract Classes 680
10.6 Case Study:Inheriting Interface and Implementation 682
10.7 Polymorphism,Virtual Functions and Dynamic Binding “Under the Hood” 695
10.8 Virtual Destructors 699
10.9 Case Study:Payroll System Using Polymorphism and Run-Time Type Information with dynamic_cast and typeid 699
11 Templates 718
11.1 Introduction 719
11.2 Function Templates 720
11.3 Overloading Function Templates 723
11.4 Class Templates 723
11.5 Class Templates and Nontype Parameters 730
11.6 Templates and Inheritance 731
11.7 Templates and Friends 731
11.8 Templates and static Members 732
12 C++ Stream Input/Output 737
12.1 Introduction 739
12.2 Streams 739
12.2.1 Classic Streams vs.Standard Streams 740
12.2.2 iostream Library Header Files 740
12.2.3 Stream Input/Output Classes and Objects 741
12.3 Stream Output 743
12.3.1 Output of char * Variables 743
12.3.2 Character Output using Member Function put 744
12.4 Stream Input 744
12.4.1 get and get line Member Functions 745
12.4.2 istream Member Functions peek,putback and ignore 748
12.4.3 Type-Safe I/O 748
12.5 Unformatted I/O using read,write and gcount 748
12.6 Introduction to Stream Manipulators 749
12.6.1 Integral Stream Base:dec,oct,hex and setbase 750
12.6.2 Floating-Point Precision (precision,setprecision) 751
12.6.3 Field Width (width,setw) 752
12.6.4 Programmer-Defined Manipulators 754
12.7 Stream Format States and Stream Manipulators 755
12.7.1 Trailing Zeros and Decimal Points (showpoint) 756
12.7.2 Justification (left,right and internal) 757
12.7.3 Padding (fill,setfill) 759
12.7.4 Integral Stream Base (dec,oct,hex,showbase) 760
12.7.5 Floating-Point Numbers; Scientific and Fixed Notation (scientific,fixed) 761
12.7.6 Uppercase/Lowercase Control (uppercase) 762
12.7.7 Specifying Boolean Format (boolalpha) 763
12.7.8 Setting and Resetting the Format State via Member-Function flags 764
12.8 Stream Error States 766
12.9 Tying an Output Stream to an Input Stream 768
13 Exception Handling 779
13.1 Introduction 780
13.2 Exception-Handling Overview 781
13.3 Other Error-Handling Techniques 783
13.4 Simple Exception-Handling Example:Divide by Zero 784
13.5 Rethrowing an Exception 788
13.6 Exception Specifications 789
13.7 Processing Unexpected Exceptions 790
13.8 Stack Unwinding 790
13.9 Constructors,Destructors and Exception Handling 792
13.10 Exceptions and Inheritance 793
13.11 Processing new Failures 793
13.12 Class auto_ptr and Dynamic Memory Allocation 797
13.13 Standard Library Exception Hierarchy 800
14 File Processing 808
14.1 Introduction 809
14.2 The Data Hierarchy 809
14.3 Files and Streams 811
14.4 Creating a Sequential-Access File 812
14.5 Reading Data from a Sequential-Access File 816
14.6 Updating Sequential-Access Files 823
14.7 Random-Access Files 824
14.8 Creating a Random-Access File 824
14.9 Writing Data Randomly to a Random-Access File 829
14.10 Reading Data Sequentially from a Random-Access File 831
14.11 Example:A Transaction-Processing Program 834
14.12 Input/Output of Objects 841
15 Class string and String Stream Processing 850
15.1 Introduction 851
15.2 string Assignment and Concatenation 852
15.3 Comparing strings 855
15.4 Substrings 857
15.5 Swapping strings 858
15.6 string Characteristics 859
15.7 Finding Strings and Characters in a string 862
15.8 Replacing Characters in a string 864
15.9 Inserting Characters into a string 866
15.10 Conversion to C-Style char * Strings 867
15.11 Iterators 869
15.12 String Stream Processing 870
16 Web Programming with CGI 880
16.1 Introduction 881
16.2 HTTP Request Types 882
16.3 Multi-Tier Architecture 882
16.4 Accessing Web Servers 883
16.5 Apache HTTP Server 884
16.6 Requesting XHTML Documents 885
16.7 Introduction to CGI 885
16.8 Simple HTTP Transaction 886
16.9 Simple CGI Script 888
16.10 Sending Input to a CGI Script 895
16.11 Using XHTML Forms to Send Input 897
16.12 Other Headers 905
16.13 Case Study:An Interactive Web Page 905
16.14 Cookies 909
16.15 Server-Side Files 915
16.16 Case Study:Shopping Cart 921
16.17 Internet and Web Resources 936
17 Data Structures 942
17.1 Introduction 943
17.2 Self-Referential Classes 944
17.3 Dynamic Memory Allocation and Data Structures 945
17.4 Linked Lists 945
17.5 Stacks 960
17.6 Queues 965
17.7 Trees 969
18 Bits,Characters,Strings and Structures 1000
18.1 Introduction 1001
18.2 Structure Definitions 1001
18.3 Initializing Structures 1003
18.4 Using Structures with Functions 1004
18.5 typedef 1004
18.6 Example:High-Performance Card-Shuffling and Dealing Simulation 1005
18.7 Bitwise Operators 1007
18.8 Bit Fields 1017
18.9 Character-Handling Library 1020
18.10 String-Conversion Functions 1026
18.11 Search Functions of the String-Handling Library 1031
18.12 Memory Functions of the String-Handling Library 1036
19 Preprocessor 1053
19.1 Introduction 1054
19.2 The #include Preprocessor Directive 1054
19.3 The #define Preprocessor Directive:Symbolic Constants 1055
19.4 The #define Preprocessor Directive:Macros 1056
19.5 Conditional Compilation 1057
19.6 The #error and #pragma Preprocessor Directives 1058
19.7 The # and ## Operators 1059
19.8 Line Numbers 1059
19.9 Predefined Symbolic Constants 1060
19.10 Assertions 1060
20 C Legacy Code Topics 1065
20.1 Introduction 1066
20.2 Redirecting Input/Output on UNIX and DOS Systems 1066
20.3 Variable-Length Argument Lists 1067
20.4 Using Command-Line Arguments 1070
20.5 Notes on Compiling Multiple-Source-File Programs 1071
20.6 Program Termination with exit and atexit 1073
20.7 The volatile Type Qualifier 1075
20.8 Suffixes for Integer and Floating-Point Constants 1075
20.9 Signal Handling 1075
20.10 Dynamic Memory Allocation with calloc and realloc 1078
20.11 The Unconditional Branch:goto 1079
20.12 Unions 1080
20.13 Linkage Specifications 1084
21 Standard Template Library (STL) 1090
21.1 Introduction to the Standard Template Library (STL) 1092
21.1.1 Introduction to Containers 1094
21.1.2 Introduction to Iterators 1098
21.1.3 Introduction to Algorithms 1103
21.2 Sequence Containers 1105
21.2.1 vector Sequence Container 1105
21.2.2 l is t Sequence Container 1113
21.2.3 deque Sequence Container 1117
21.3 Associative Containers 1119
21.3.1 multiset Associative Container 1119
21.3.2 set Associative Container 1122
21.3.3 mu l t imap Associative Container 1124
21.3.4 map Associative Container 1126
21.4 Container Adapters 1128
21.4.1 stack Adapter 1128
21.4.2 queue Adapter 1130
21.4.3 priority_queue Adapter 1132
21.5 Algorithms 1133
21.5.1 fill,fill_n,generate and generate_n 1134
21.5.2 equal,mismatch and lexicographical_compare 1136
21.5.3 remove,remove_if,remove_copy and remove_copy_if 1138
21.5.4 replace,replace_if,replace_copy and replace_copy_if 1141
21.5.5 Mathematical Algorithms 1144
21.5.6 Basic Searching and Sorting Algorithms 1148
21.5.7 swap,iter_swap and swap_ranges 1150
21.5.8 copy_backward,merge,unique and reverse 1152
21.5.9 inplace_merge,unique_copy and reverse_copy 1154
21.5.10 Set Operations 1156
21.5.11 lower_bound,upper_bound and equal_range 1160
21.5.12 Heapsort 1162
21.5.13 min and max 1165
21.5.14 Algorithms Not Covered in This Chapter 1166
21.6 Class bitset 1168
21.7 Function Objects 1172
21.8 STL Internet and Web Resources 1175
22 Other Topics 1183
22.1 Introduction 1184
22.2 const_cast Operator 1184
22.3 reinterpret_cast Operator 1185
22.4 namespaces 1186
22.5 Operator Keywords 1190
22.6 explicit Constructors 1192
22.7 mutable Class Members 1197
22.8 Pointers to Class Members (.* and ->*) 1199
22.9 Multiple Inheritance 1201
22.10 Multiple Inheritance and virtual Base Classes 1205
22.11 Closing Remarks 1210
A Operator Precedence Chart 1214
B ASCII Character Set 1216
C Number Systems 1217
C.1 Introduction 1218
C.2 Abbreviating Binary Numbers as Octal Numbers and Hexadecimal Numbers 1221
C.3 Converting Octal Numbers and Hexadecimal Numbers to Binary Numbers 1222
C.4 Converting from Binary,Octal or Hexadecimal to Decimal 1222
C.5 Converting from Decimal to Binary,Octal or Hexadecimal 1223
C.6 Negative Binary Numbers:Two’s Complement Notation 1225
D C++ Internet and Web Resources 1230
D.1 Resources 1230
D.2 Tutorials 1232
D.3 FAQs 1233
D.4 Visual C++ 1233
D.5 Newsgroups 1233
D.6 Compilers and Development Tools 1234
D.7 Standard Template Library 1234
E Introduction to XHTML 1236
E.1 Introduction 1237
E.2 Editing XHTML 1237
E.3 First XHTML Example 1238
E.4 Headers 1240
E.5 Linking 1242
E.6 Images 1245
E.7 Special Characters and More Line Breaks 1249
E.8 Unordered Lists 1251
E.9 Nested and Ordered Lists 1251
E.10 Basic XHTML Tables 1252
E.11 Intermediate XHTML Tables and Formatting 1257
E.12 Basic XHTML Forms 1259
E.13 More Complex XHTML Forms 1262
E.14 Internet and World Wide Web Resources 1269
F XHTML Special Characters 1274
Bibliography 1275
Index 1281
- 《高级英语阅读与听说教程》刘秀梅编著 2019
- 《大学计算机实验指导及习题解答》曹成志,宋长龙 2019
- 《看图自学吉他弹唱教程》陈飞编著 2019
- 《激光加工实训技能指导理实一体化教程 下》王秀军,徐永红主编;刘波,刘克生副主编 2017
- 《AutoCAD 2019 循序渐进教程》雷焕平,吴昌松,陈兴奎主编 2019
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《Photoshop CC 2018基础教程》温培利,付华编著 2019
- 《大学生心理健康与人生发展》王琳责任编辑;(中国)肖宇 2019
- 《剑桥国际英语写作教程 段落写作》(美)吉尔·辛格尔顿(Jill Shingleton)编著 2019
- 《大学英语四级考试全真试题 标准模拟 四级》汪开虎主编 2012
- 《高等数学试题与详解》西安电子科技大学高等数学教学团队 2019
- 《重庆市绿色建筑评价技术指南》重庆大学,重庆市建筑节能协会绿色建筑专业委员会主编 2018
- 《刘泽华全集 先秦政治思想史 下》刘泽华著;南开大学历史学院编 2019
- 《高等数学 上》东华大学应用数学系编 2019
- 《江苏中小企业生态环境评价报告》南京大学金陵学院企业生态研究中心 2019
- 《国学 第6集》四川师范大学中华传统文化学院四川省人民政府文史研究馆 2018
- 《西中有东》华大学国学院编;(美)包华石;王金凤译 2019
- 《江苏中小企业生态环境评价报告 2016》南京大学金陵学院企业生态研究中心 2017
- 《江苏中小企业生态环境评价报告 2017》南京大学金陵学院企业生态研究中心 2018
- 《闻声知道 古琴文化读本》厦门大学汉语国际推广南方基地,龙人古琴研究院 2018
- 《“中国印”的世界 骆芃芃篆刻书法艺术展作品集 the collection of exhibition of seal engraving and calligraphic works》骆芃芃著 2012
- 《Foundations of criminal law》[edited] by Leo Katz 1999
- 《DETECTION AND SPECTROMETRY OF FAINT LIGHT》JOHN MEABURN
- 《冷战后美日同盟转型研究=STUDY ON TRANSFORMATION OF THE US-JAPAN ALLIANCE AFTER THE COLD WAR》衣昭杰著 2020
- 《现代艺术的源代码=THE SHORT STORY OF MODERN ART》(英)苏西·霍奇著 2020
- 《唐帝国的香气=A BRIEF HISTORY OF THE TANG DYNASTY》殷靖著 2020
- 《幸福感符号学 社会文化修辞=SEMIOTICS OF HAPPINESS RHETORIC BEGINNING OF A PUBLIC PROBLEM》(英)阿什利·弗劳利(Ashley Frawley)著 2019
- 《细节中的新中国史=THE HISTORY OF THE PEOPLES REPUBLIC OF CHINA IN DETAILS》张神根 2020
- 《汉译文学性概论=RESEARCH ON LITERARINESS OF FOREIGN LITERATURE TRANSLATION INTO CHINESE》侯影著 2019
- 《燃料电池汽车动力系统分布式测试数据传输研究=DATA TRANSMISSION ANALYSIS OF DISTRIBUTED TEST PLATFORM FOR FUEL CELL》牛文旭著 2020