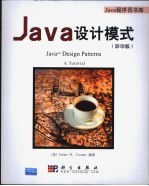
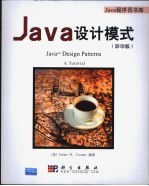
JAVA设计模式PDF电子书下载
- 电子书积分:12 积分如何计算积分?
- 作 者:(美)库珀(Cooper,J.W.)编著
- 出 版 社:北京:科学出版社
- 出版年份:2004
- ISBN:7030124944
- 页数:329 页
Section 1 What Are Design Patterns? 1
Chapter 1 Introduction 3
Defining Design Patterns 5
The Learning Process 6
Studying Design Patterns 7
Notes on Object-Oriented Approaches 7
The Java Foundation Classes 8
Java Design Patterns 9
Chapter 2 UML Diagrams 11
Inheritance 12
Interfaces 13
CONTENTSPreface 13
Composition 14
Acknowledgments 15
Annotation 15
JVISION UML Diagrams 15
Visual SlickEdit Project Files 15
Section 2 Creational Patterns 17
Chapter 3 The Factory Pattern 19
How a Factory Works 19
Sample Code 20
The Two Subclasses 20
Building the Simple Factory 21
Factory Patterns in Math Computation 23
Thought Questions 24
Programs on the CD-ROM 24
Chapter 4 The Factory Method 25
The Swimmer Class 27
The Event Classes 27
Straight Seeding 28
Our Seeding Program 30
Other Factories 30
When to Use a Factory Method 31
Thought Question 31
Programs on the CD-ROM 31
Chapter 5 The Abstract Factory Pattern 33
A GardenMaker Factory 34
How the User Interface Works 36
Adding More Classes 37
Consequences of the Abstract Factory Pattern 37
Thought Question 38
Programs on the CD-ROM 38
Chapter 6 The Singleton Pattern 39
Creating a Singleton Using a Static Method 39
Exceptions and Instances 40
Throwing an Exception 41
Creating an Instance of the Class 41
Providing a Global Point of Access to a Singleton Pattern 42
The javax.comm Package as a Singleton 43
Other Consequences of the Singleton Pattern 46
Thought Question 47
Programs on the CD-ROM 47
Chapter 7 The Builder Pattern 49
An Investment Tracker 50
Calling the Builders 52
The List Box Builder 54
The Check Box Builder 54
Consequences of the Builder Pattern 55
Thought Questions 56
Programs on the CD-ROM 56
Chapter 8 The Prototype Pattern 57
Using the Prototype 58
Cloning in Java 58
Using the Prototype Pattern 61
Prototype Managers 64
Cloning Using Serialization 65
Consequences of the Prototype Pattern 66
Thought Question 66
Programs on the CD-ROM 67
Summary of Creational Patterns 67
Section 3 Structural Patterns 69
Moving Data between Lists 71
Chapter 9 The Adapter Pattern 71
Using the JFC JList Class 73
Two-Way Adapters 78
Pluggable Adapters 78
Adapters in Java 79
Thought Question 81
Programs on the CD-ROM 81
Chapter 10 The Bridge Pattern 83
The Class Diagram 85
Extending the Bridge 86
Java Beans as Bridges 87
Consequences of the Bridge Pattern 88
Thought Question 89
Programs on the CD-ROM 89
Chapter 11 The Composite Pattern 91
An Implementation of a Composite 92
Computing Salaries 93
The Employee Classes 93
The Boss Class 95
Building the Employee Tree 97
Self-Promotion 98
Doubly Linked List 99
Consequences of the Composite Pattern 100
A Simple Composite 100
Composites in Java 100
Thought Questions 101
Programs on the CD-ROM 101
Other Implementation Issues 101
Chapter 12 The Decorator Pattern 103
Decorating a CoolButton 103
Using a Decorator 105
The Class Diagram 107
Decorating Borders in Java 108
Nonvisual Decorators 109
Decorators, Adapters, and Composites 112
Programs on the CD-ROM 113
Consequences of the Decorator Pattern 113
Thought Questions 113
Chapter 13 The Fa?ade Pattern 115
Building the Fa?ade Classes 117
Consequences of the Fa?ade Pattern 120
Notes on Installing and Running the dbFrame Program 121
Thought Question 122
Programs on the CD-ROM 122
Chapter 14 The Flyweight Pattern 123
Discussion 124
Example Code 124
Flyweight Uses in Java 129
Sharable Objects 130
Copy-on-Write Objects 130
Thought Question 131
Programs on the CD-ROM 131
Chapter 15 The Proxy Pattern 133
Sample Code 134
Copy-on-Write 136
Enterprise Java Beans 136
Comparison with Related Patterns 137
Thought Question 137
Programs on the CD-ROM 137
Summary of Structural Patterns 137
Section 4 Behavioral Patterns 139
Chapter 16 Chain of Responsibility Pattern 141
Applicability 142
Sample Code 143
The List Boxes 145
Programming a Help System 148
A Chain or a Tree? 151
Kinds of Requests 152
Examples in Java 152
Consequences of the Chain of Responsibility 153
Thought Questions 153
Programs on the CD-ROM 154
Chapter 17 The Command Pattern 155
Motivation 155
Command Objects 156
Building Command Objects 157
The Command Pattern 159
The Command Pattern in the Java Language 161
Consequences of the Command Pattern 162
Providing Undo 163
Thought Questions 167
Programs on the CD-ROM 168
Chapter 18 The Interpreter Pattern 169
Motivation 169
Applicability 170
Simple Report Example 170
Interpreting the Language 171
Objects Used in Parsing 172
Reducing the Parsed Stack 175
Implementing the Interpreter Pattern 176
Consequences of the Interpreter Pattern 180
Thought Question 181
Programs on the CD-ROM 181
Chapter 19 The Iterator Pattern 183
Motivation 183
Enumerations in Java 184
Sample Code 184
Filtered Iterators 185
Consequence of the Iterator Pattern 188
Composites and Iterators 189
Iterators in Java 1.2 189
Thought Question 189
Programs on the CD-ROM 190
Chapter 20 The Mediator Pattern 191
An Example System 191
Interactions between Controls 193
Sample Code 194
Mediators and Command Objects 197
Consequences of the Mediator Pattern 198
Single Interface Mediators 198
Implementation Issues 199
Programs on the CD-ROM 199
Chapter 21 The Memento Pattern 201
Motivation 201
Implementation 202
Sample Code 203
Consequences of the Memento Pattern 207
Thought Question 208
Programs on the CD-ROM 208
Chapter 22 The Observer Pattern 209
Watching Colors Change 210
The Message to the Media 213
The JList as an Observer 214
Consequences of the Observer Pattern 216
The Observer Interface and Observable Class 216
The MVC Architecture as an Observer 216
Thought Questions 217
Programs on the CD-ROM 217
Chapter 23 The State Pattern 219
Sample Code 219
Switching between States 224
How the Mediator Interacts with the StateManager 225
Consequences of the State Pattern 227
Mediators and the God Class 227
State Transitions 227
Thought Questions 228
Programs on the CD-ROM 228
Chapter 24 The Strategy Pattern 229
Motivation 229
Sample Code 230
The Context Class 231
The Program Commands 232
Drawing Plots in Java 233
The Line and Bar Graph Strategies 233
Consequences of the Strategy Pattern 236
Thought Question 237
Programs on the CD-ROM 237
Chapter 25 The Template Pattern 239
Motivation 239
Kinds of Methods in a Template Class 241
Template Method Patterns in Java 241
Sample Code 242
Templates and Callbacks 246
Consequences of the Template Pattern 247
Thought Question 247
Programs on the CD-ROM 247
Chapter 26 The Visitor Pattern 249
Motivation 249
When to Use the Visitor Pattern 251
Sample Code 251
Visiting Several Classes 253
Visiting the Classes 253
Bosses are Employees, Too 255
Catch-All Operations Using Visitors 256
Double Dispatching 257
Traversing a Series of Classes 257
Consequence of the Visitor Pattern 257
Thought Question 258
Programs on the CD-ROM 258
Section 5 Design Patterns and the Java Foundation Classes 259
Chapter 27 The JFC, or Swing 261
Installing and Using Swing 261
Ideas behind Swing 262
The Swing Class Hierarchy 262
Chapter 28 Writing a Simple JFC Program 263
Setting the Look and Feel 263
Setting the Window Close Box 264
Making a JxFrame Class 264
A Simple Two-Button Program 265
More on JButton 266
Programs on the CD-ROM 267
Chapter 29 Radio Buttons and Toolbars 269
Radio Buttons 269
The JToolBar 270
JToggleButton 270
A Sample Button Program 271
Programs on the CD-ROM 271
Action Objects 273
Chapter 30 Menus and Actions 273
Design Patterns in the Action Object 276
Programs on the CD-ROM 277
Chapter 31 The JList Class 279
List Selections and Events 280
Changing a List Display Dynamically 281
A Sorted JList with a ListModel 282
Sortirg More-Complicated Objects 284
Getting Database Keys 286
Adding Pictures in List Boxes 288
Programs on the CD-ROM 289
Chapter 32 The JTable Class 291
A Simple JTable Program 291
Cell Renderers 295
Rendering Other Kinds of Classes 296
Selecting Cells in a Table 298
Patterns Used in This Image Table 299
Programs on the CD-ROM 301
Chapter 33 The JTree Class 303
The TreeModel Interface 305
Programs on the CD-ROM 305
Summary 305
Section 6 Case Studies 307
Chapter 34 Sandy and the Mediator 309
Chapter 35 Herb'sText ProcessingTangle 313
Chapter 36 Mary's Dilemma 315
Bibliography 317
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《设计十六日 国内外美术院校报考攻略》沈海泯著 2018
- 《计算机辅助平面设计》吴轶博主编 2019
- 《高校转型发展系列教材 素描基础与设计》施猛责任编辑;(中国)魏伏一,徐红 2019
- 《景观艺术设计》林春水,马俊 2019
- 《高等教育双机械基础课程系列教材 高等学校教材 机械设计课程设计手册 第5版》吴宗泽,罗圣国,高志,李威 2018
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019
- 《Cinema 4D电商美工与视觉设计案例教程》樊斌 2019
- 《通信电子电路原理及仿真设计》叶建芳 2019
- 《高等学校“十三五”规划教材 C语言程序设计》翟玉峰责任编辑;(中国)李聪,曾志华,江伟 2019
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《《走近科学》精选丛书 中国UFO悬案调查》郭之文 2019
- 《北京生态环境保护》《北京环境保护丛书》编委会编著 2018
- 《中医骨伤科学》赵文海,张俐,温建民著 2017
- 《美国小学分级阅读 二级D 地球科学&物质科学》本书编委会 2016
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019
- 《强磁场下的基础科学问题》中国科学院编 2020
- 《小牛顿科学故事馆 进化论的故事》小牛顿科学教育公司编辑团队 2018
- 《小牛顿科学故事馆 医学的故事》小牛顿科学教育公司编辑团队 2018
- 《高等院校旅游专业系列教材 旅游企业岗位培训系列教材 新编北京导游英语》杨昆,鄢莉,谭明华 2019