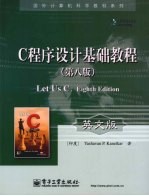
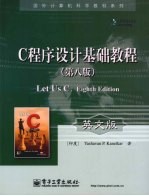
C程序设计基础教程 英文版PDF电子书下载
- 电子书积分:14 积分如何计算积分?
- 作 者:亚沙万P·卡内特卡编著
- 出 版 社:北京:电子工业出版社
- 出版年份:2009
- ISBN:9787121092671
- 页数:449 页
Chapter 1:Getting Started 1
1.1 Whatis C 2
1.2 Getting Started with C 3
1.2.1 The C Character Set 3
1.2.2 Constants,Variables and Keywords 4
1.2.3 Types of C Constants 4
1.2.4 Rules for Constructing Integer Constants 5
1.2.5 Rules for Constructing Real Constants 5
1.2.6 Rules for Constructing Character Constants 6
1.2.7 Types of C Variables 6
1.2.8 Rules for Constructing Variable Names 7
1.2.9 C Keywords 7
1.3 The First C Program 8
1.4 Compilation and Execution 11
1.5 Receiving Input 12
1.6 C Instructions 14
1.6.1 Type Declaration Instruction 14
1.6.2 Arithmetic Instruction 15
1.6.3 Integer and Float Conversions 17
1.6.4 Type Conversion in Assignments 17
1.6.5 Hierarchy of Operations 19
1.6.6 Associativity of Operators 20
1.7 Control Instructions in C 22
1.8 Summary 22
1.9 Exercise 23
Chapter 2:The Decision Control Structure 30
2.1 Decisions!Decisions! 30
2.2 The if Statement 31
2.2.1 The Real Thing 33
2.2.2 Multiple Statements within if 34
2.3 The if-else Statement 35
2.3.1 Nested if-elses 37
2.3.2 Forms of if 38
2.4 Use of Logical Operators 39
2.4.1 The else if Clause 41
2.4.2 The!Operator 44
2.4.3 Hierarchy of Operators Revisited 45
2.5 A Word of Caution 45
2.6 The Conditional Operators 47
2.7 Summary 48
2.8 Exercise 48
Chapter3:The Loop Control Structure 60
3.1 Loops 60
3.2 The while Loop 61
3.2.1 Tips and Traps 62
3.2.2 More Operators 65
3.3 The for Loop 67
3.3.1 Nesting of Loops 70
3.3.2 Multiple lnitialisations in the for Loop 71
3.4 The Odd Loop 72
3.5 The break Statement 73
3.6 The continue Statement 74
3.7 The do-while Loop 75
3.8 Summary 76
3.9 Exercise 77
Chapter4:The Case Control Structurs 84
4.1 Decisions Using switch 84
4.1.1 The Tips and Traps 87
4.2 switch Versus if-else Ladder 90
4.3 The goto Keyword 90
4.4 Summary 92
4.5 Exercise 92
Chapter 5:Functions & Pointers 97
5.1 What is a Function 97
5.1.1 Why Use Functions 102
5.2 Passing Values between Functions 103
5.3 Scope Rule of Functions 106
5.4 Calling Convention 106
5.5 One Dicey Issue 107
5.6 Advanced Features of Functions 108
5.6.1 Return Type of Function 108
5.6.2 Call by Value and Call by Reference 109
5.6.3 An Introduction to Pointers 109
5.6.4 Pointer Notation 109
5.6.5 Back to Function Calls 113
5.6.6 Conclusions 115
5.6.7 Recursion 116
5.6.8 Recursion and Stack 119
5.7 Adding Functions to the Library 120
5.8 Summary 122
5.9 Exercise 123
Chapter6:Data Types Revisited 132
6.1 Integers,long and short 132
6.2 Integers,signed and unsigned 134
6.3 Chars,signed and unsigned 134
6.4 Floats and Doubles 135
6.5 A Few More Issues 137
6.6 Storage Classes in C 138
6.6.1 Automatic Storage Class 138
6.6.2 Register Storage Class 140
6.6.3 Static Storage Class 141
6.6.4 External Storage Class 142
6.6.5 A Few Subtle Issues 144
6.6.6 Which to Use When 145
6.7 Summary 146
6.8 Exercise 146
Chapter 7:The C Preprocessor 152
7.1 Features of C Preprocessor 152
7.2 Macro Expansion 153
7.2.1 Macros with Arguments 155
7.2.2 Macros versus Functions 158
7.3 File Inclusion 158
7.4 Conditional Compilation 159
7.5 #if and #elif Directives 162
7.6 Miscellaneous Directives 163
7.6.1 #undef Directive 163
7.6.2 #pragma Directive 163
7.7 The Build Process 165
7.7.1 Preprocessing 166
7.7.2 Compilation 166
7.7.3 Assembling 166
7.7.4 Linking 167
7.7.5 Loading 168
7.8 Summary 168
7.9 Exercise 169
Chapter 8:Arrays 172
8.1 What are Arrays 172
8.1.1 A Simple Program Using Array 173
8.2 More on Arrays 175
8.2.1 Array Initialisation 175
8.2.2 Bounds Checking 176
8.2.3 Passing Array Elements to a Function 177
8.3 Pointers and Arrays 178
8.3.1 Passing an Entire Array to a Function 183
8.3.2 The Real Thing 183
8.4 Two Dimensional Arrays 184
8.4.1 Initialising a 2-Dimensional Array 185
8.4.2 Memory Map of a 2-Dimensional Array 186
8.4.3 Pointers and 2-Dimensional Arrays 186
8.4.4 Pointer to an Array 188
8.4.5 Passing 2-D Array to a Function 189
8.5 Array of Pointers 192
8.6 Three-Dimensional Array 193
8.7 Summary 194
8.8 Exercise 195
Chapter 9:Puppetting On Strings 210
9.1 What are Strings 210
9.2 More about Strings 211
9.3 Pointers and Strings 214
9.4 Standard Library String Functions 215
9.4.1 strlen() 215
9.4.2 strcpy() 217
9.4.3 strcat() 219
9.4.4 strcmp() 219
9.5 Two-Dimensional Array of Characters 220
9.6 Array of Pointers to Strings 222
9.7 Limitation of Array of Pointers to Strings 224
9.7.1 Solution 225
9.8 Summary 226
9.9 Exercise 226
Chapter 10:Structures 231
10.1 Why Use Structures 231
10.1.1 Declaring a Structure 233
10.1.2 Accessing Structure Elements 235
10.1.3 How Structure Elements are Stored 235
10.2 Array of Structures 236
10.3 Additional Features of Structures 237
10.4 Uses of Structures 243
10.5 Summary 244
10.6 Exercise 244
Chapter 11:Console Input/Output 249
11.1 Types of I/O 249
11.2 Console I/O Functions 250
11.2.1 Formatted Console I/O Functions 250
11.2.2 sprintf() and sscanf() Functions 256
11.2.3 Unformatted Console I/O Functions 256
11.3 Summary 259
11.4 Exercise 259
Chapter 12:File Input/Output 263
12.1 Data Organization 264
12.2 File Operations 264
12.2.1 Opening a File 265
12.2.2 Reading from a File 266
12.2.3 Trouble in Opening a File 266
12.2.4 Closing the File 267
12.3 Counting Characters,Tabs,Spaces, 268
12.4 A File-copy Program 269
12.4.1 Wriring to a File 269
12.5 File Opening Modes 270
12.6 Siring(line)I/O in Files 270
12.6.1 The Awkward Newline 272
12.7 Record I/O in Files 272
12.8 Text Files and Binary Files 275
12.9 Record I/O Revisited 277
12.10 Database Management 279
12.11 Low Level Disk I/O 283
12.11.1 A Low Level File-copy Program 283
12.12 I/O Under Windows 286
12.13 Summary 286
12.14 Exercise 287
Chapter 13:More Issues In Input/Output 294
13.1 Using argc and argv 294
13.2 Detecting Errors in Reading/Writing 297
13.3 Standard I/O Devices 298
13.4 I/O Redirection 299
13.4.1 Redirecting the Output 299
13.4.2 Redirecting the Input 300
13.4.3 Both Ways at Once 301
13.5 Summary 301
13.6 Exercise 302
Chapter 14:Operations On Bits 303
14.1 Bitwise Operators 303
14.1.1 One's Complement Operator 305
14.1.2 Right Shift Operator 306
14.1.3 Left Shift Operator 307
14.1.4 Bitwise AND Operator 310
14.1.5 Bitwise OR Operator 313
14.1.6 Bitwise XOR Operator 313
14.2 The showbits() Function 314
14.3 Hexadecimal Numbering System 315
14.4 Relation between Binary and Hex 316
14.5 Summary 317
14.6 Exercise 317
Chapter 15:Miscellaneous Features 320
15.1 Enumerated Data Type 320
15.1.1 Uses of Enumerated Data Type 321
15.1.2 Are Enums Necessary 323
15.2 Renaming Data types with typedef 323
15.3 Typecasting 325
15.4 Bit Fields 326
15.5 Pointers to Functions 327
15.6 Functions Returning Pointers 329
15.7 Functions with Variable Number of Arguments 330
15.8 Unions 332
15.8.1 Union of Structures 336
15.8.2 Utility of Unions 337
15.9 The volatile Qualifier 338
15.10 Summary 339
15.11 Exercise 339
Chapter 16:C Under Windows 342
16.1 Which Windows 343
16.2 Salient Features of Windows Programming 343
16.2.1 Powerful API Functions 344
16.2.2 Sharing of Functions 344
16.2.3 Consistent Look and Feel 344
16.2.4 Hardware Independent Programming 345
16.2.5 Event Driven Programming Model 345
16.3 Obvious Programming Differences 347
16.3.1 Integers 347
16.3.2 Heavy Use of typedef 347
16.3.3 Size of Pointers 348
16.4 The First Windows Program 349
16.5 Hungarian Notation 351
16.6 Role of the Message Box 351
16.7 Here Comes the window 352
16.8 More Windows 354
16.9 A Real-World Window 355
16.9.1 Creation and Displaying of Window 356
16.9.2 Interaction with Window 356
16.9.3 Reacting to Messages 357
16.10 Program Instances 359
16.11 Summary 359
16.12 Exercise 360
Chapter 17:Graphics Under Windows 361
17.1 Graphics as of Now 361
17.2 Device Independent Drawing 362
17.3 Hello Windows 362
17.4 Drawing Shapes 365
17.5 Types of Pens 367
17.6 Types of Brushes 369
17.6.1 Code and Resources 371
17.7 Freehand Drawing,the Paintbrush Style 371
17.7.1 Capturing the Mouse 373
17.8 Device Context,a Closer Look 374
17.9 Displaying a Bitmap 375
17.10 Animation at Work 377
17.10.1 WM_CREATE and OnCreate() 379
17.10.2 WM_TIMER and OnTimer() 380
17.10.3 A Few More Points 380
17.11 Windows.the Endless World 381
17.12 Summary 381
17.13 Exercise 382
Chapter 18:Internet Programming 383
18.1 Network Communication 383
18.2 Packets and Sockets 385
18.3 Before We Start 385
18.3.1 Protocols 385
18.3.2 IP Addresses 386
18.3.3 Port Numbers 386
18.3.4 Byte Ordering 387
18.4 Getting Started 387
18.5 What's The Time Now 389
18.5.1 Creation of Socket 392
18.5.2 Sending Data to a Time Server 392
18.5.3 Receiving Date and Time 393
18.6 Communicating with Whois Server 393
18.7 Give Me the Home Page 396
18.8 Sending and Receiving Emails 397
18.9 Two-Way Communication 405
18.10 Summary 410
18.11 Exercise 410
Chapter 19:C Under Linux 411
19.1 What is Linux 411
19.2 C Programming Under Linux 412
19.3 The'Hello Linux'Program 412
19.4 Processes 413
19.5 Parent and Child Processes 414
19.6 More Processes 417
19.7 Zombies and Orphans 418
19.8 One Interesting Fact 419
19.9 Summary 420
19.10 Exercise 420
Chapter 20:More Linux Programming 422
20.1 Communication using Signals 422
20.2 Handling Multiple Signals 424
20.3 Registering a Common Handler 425
20.4 Blocking Signals 426
20.5 Event Driven Programming 428
20.6 Where Do You Go From Here 431
20.7 Summary 431
20.8 Exercise 432
Appendix A:Precedence Table 433
Appendix B:Library Functions 434
Appendix C:Chasing The Bugs 440
Appendix D:ASCII Chart 444
Appendix E:Helper.h File 447
Appendix F:Linux Installation 448
- 《市政工程基础》杨岚编著 2009
- 《零基础学会素描》王金著 2019
- 《计算机网络与通信基础》谢雨飞,田启川编著 2019
- 《生物质甘油共气化制氢基础研究》赵丽霞 2019
- 《花时间 我的第一堂花艺课 插花基础技法篇》(日)花时间编辑部编;陈洁责编;冯莹莹译 2020
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《Photoshop CC 2018基础教程》温培利,付华编著 2019
- 《看视频零基础学英语口语》宋德伟 2019
- 《设计十六日 国内外美术院校报考攻略》沈海泯著 2018
- 《胃癌基础病理》(日)塚本彻哉编者;宫健,刘石译者 2019
- 《市政工程基础》杨岚编著 2009
- 《家畜百宝 猪、牛、羊、鸡的综合利用》山西省商业厅组织技术处编著 1959
- 《《道德经》200句》崇贤书院编著 2018
- 《高级英语阅读与听说教程》刘秀梅编著 2019
- 《计算机网络与通信基础》谢雨飞,田启川编著 2019
- 《看图自学吉他弹唱教程》陈飞编著 2019
- 《法语词汇认知联想记忆法》刘莲编著 2020
- 《培智学校义务教育实验教科书教师教学用书 生活适应 二年级 上》人民教育出版社,课程教材研究所,特殊教育课程教材研究中心编著 2019
- 《国家社科基金项目申报规范 技巧与案例 第3版 2020》文传浩,夏宇编著 2019
- 《流体力学》张扬军,彭杰,诸葛伟林编著 2019
- 《电子测量与仪器》人力资源和社会保障部教材办公室组织编写 2009
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《北京生态环境保护》《北京环境保护丛书》编委会编著 2018
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019
- 《通信电子电路原理及仿真设计》叶建芳 2019
- 《高等院校旅游专业系列教材 旅游企业岗位培训系列教材 新编北京导游英语》杨昆,鄢莉,谭明华 2019
- 《电子应用技术项目教程 第3版》王彰云 2019
- 《中国十大出版家》王震,贺越明著 1991
- 《近代民营出版机构的英语函授教育 以“商务、中华、开明”函授学校为个案 1915年-1946年版》丁伟 2017