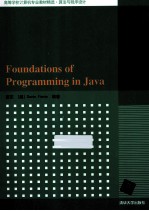
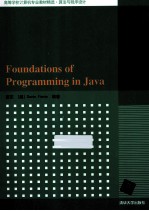
Java基础教程 英文PDF电子书下载
- 电子书积分:14 积分如何计算积分?
- 作 者:董东,(澳)芬尼编著
- 出 版 社:北京:清华大学出版社
- 出版年份:2013
- ISBN:9787302325512
- 页数:408 页
Chapter 1 Introduction to Java Programming 1
1.1 Abstraction 1
1.2 Development Environment and Running Environment 3
1.3 Programming in the Command Prompt Window 4
1.4 Programming in Eclipse 7
1.5 Java Application Structure 11
1.6 Code Style 12
1.6.1 Naming Conventions 13
1.6.2 Indentation and Spacing 13
1.6.3 Block Styles 14
1.7 Comments 15
1.8 Java and Development Tools 17
1.9 Foundations of Object-Oriented Programming 17
1.10 New Terminology 19
1.11 NewWords and Expressions 21
1.12 Hands on Lab 21
1.12.1 Installing JDK and Eclipse IDE 21
1.12.2 Programming in Command Prompt Window 22
1.12.3 Programming in Eclipse 24
1.12.4 Exporting and Importing Java Projects in Eclipse 28
1.13 Exercises 32
Chapter 2 The Basics of Java Language 33
2.1 Identifiers 33
2.2 Primitive Data Types 34
2.3 Literals 36
2.4 Variables 37
2.5 Operators 40
2.5.1 Assignment 40
2.5.2 Arithmetic Operators 41
2.5.3 Relational Operators 41
2.5.4 Logical Operators 42
2.5.5 Bitwise Operators 43
2.5.6 Conditional Operator 43
2.5.7 Operator Precedence 44
2.6 Expressions and Statements 45
2.7 The Scanner Class 46
2.8 Control Structures 48
2.8.1 Sequence Structures 49
2.8.2 Selection Structures 49
2.8.3 Repetition Structures 53
2.8.4 Branching Statements 56
2.9 Arrays 57
2.10 Built-in Java Classes 61
2.10.1 Java Strings 61
2.10.2 The StringBuffer Class 66
2.10.3 Random Numbers 67
2.10.4 BigInteger Objects 68
2.10.5 Date and Time 68
2.10.6 Wrapper Classes 71
2.11 Command Line Arguments 73
2.12 New Terminology 74
2.13 New Words and Expressions 75
2.14 Hands on Lab 76
2.14.1 Getting Input from Keyboard via ScannerClass 76
2.14.2 Converting String Type into int Type 77
2.14.3 Two-Dimensional Array of int 79
2.14.4 Java Strings 80
2.15 Exercises 81
Chapter 3 Classes and Objects 87
3.1 Class Declaration 87
3.2 Creating Objects 94
3.3 Accessing Objects via Reference Variables 94
3.4 Object Reference this 95
3.5 Parameter Passing 96
3.6 Returning from a Method 102
3.7 Method Overloading 103
3.8 Class Variables and Instance Variables 104
3.9 Class Methods and Instance Methods 106
3.10 The Scope of Variables 108
3.11 Garbage Collection 110
3.12 Reflection 110
3.13 Code Organization 112
3.13.1 Java API 113
3.13.2 Package Customs 113
3.14 Pattern Matching with Regular Expressions 115
3.15 Summary of Creating and Using Classes and Objects 124
3.16 New Terminology 124
3.17 New Words and Expressions 126
3.18 Hands on Lab 126
3.18.1 Static Variables and Instance Variables 126
3.18.2 Static Methods and Instance Methods 127
3.19 Exercises 128
Chapter 4 Inheritance,Interface and Polymorphism 136
4.1 The Concept of Inheritance 136
4.2 Constructors of Superclass and Subclass 140
4.3 Method Overriding 142
4.4 Upcasting and Downcasting 144
4.5 Abstract Class and Abstract Method 146
4.6 Interfaces 148
4.7 Polymorphism 153
4.8 Final Classes and Final Members 154
4.9 Access Control 156
4.10 Methods in the Object Class 160
4.10.1 The toString Method 161
4.10.2 The equals Method 161
4.10.3 The hashCode Method 163
4.10.4 The clone Method 164
4.11 Comparison of Objects 169
4.12 New Terminology 172
4.13 New Words and Expressions 172
4.14 Hands on Lab 172
4.14.1 Reuse Class via Inheritance 172
4.14.2 Constructor Calling Chain 173
4.14.3 Overriding Method 174
4.14.4 Runtime Polymorphism 175
4.14.5 Interface 175
4.14.6 Access Control 177
4.15 Exercises 177
Chapter 5 Exception Handling 186
5.1 Introduction 186
5.2 Handling Exceptions 189
5.3 The Finally Block 192
5.4 User-defined Exceptions 195
5.5 Benefits of Java Exception Handling Framework 197
5.6 Assertions 200
5.7 New Terminology 203
5.8 New Words and Expressions 203
5.9 Hands on Lab 203
5.9.1 Classpath and ClassNotFoundException 203
5.9.2 Catch a Runtime Exception 206
5.9.3 Create Your Own Exception Class 207
5.10 Exercises 207
Chapter 6 Collections Framework 213
6.1 Introduction 213
6.2 Set Interface 217
6.3 List Interface 222
6.3.1 ArrayList 223
6.3.2 Algorithms for List 227
6.4 Stack 231
6.5 Queue Interface 235
6.6 Map Interface 236
6.7 Generics 240
6.8 New Terminology 245
6.9 New Words and Expressions 245
6.10 Hands on Lab 246
6.10.1 View JDK Source Code in Eclipse 246
6.10.2 Using HashSet and TreeSet 247
6.10.3 Using List 249
6.10.4 Using Map 250
6.10.5 Sorting and Searching 250
6.11 Exercises 251
Chapter 7 Stream I/O 256
7.1 Manipulating Disk Files and Folders 256
7.2 Streams 260
7.2.1 Byte Streams 261
7.2.2 Buffered Byte Streams 264
7.2.3 Data Streams 265
7.2.4 Character Streams 269
7.2.5 Buffered Character-based I/O 271
7.3 Reading Values ofVarious Types by Scanner 273
7.4 Formatted-Text Printing with printf() Method 278
7.5 Object Serialization 283
7.6 Standard I/O and Redirection 285
7.7 Character Sets and Unicode 286
7.8 New Terminology 288
7.9 New Words and Expressions 289
7.10 Hands on Lab 289
7.10.1 Buffered Stream 289
7.10.2 DataInputStream and Data OutputStream 290
7.10.3 Character Stream 290
7.10.4 printf() Method 293
7.10.5 Object Serialization 293
7.10.6 Random Access File 293
7.11 Exercises 294
Chapter 8 Creating a GUI with JFC/Swing 299
8.1 Introduction 299
8.2 Using Swing APIs and Layout Managers 299
8.3 Swing Components 303
8.4 Component Inclusion Relationship in a GUI Application 306
8.5 Dialogs 308
8.6 Layout Management 312
8.7 Menus 317
8.8 Basic Components and Their Events 320
8.9 Events 322
8.10 MVC 331
8.11 New Terminology 339
8.12 New Words and Expressions 339
8.13 Hands on Lab 340
8.13.1 JButton 340
8.13.2 Action Event 340
8.13.3 Dialogs 340
8.13.4 Layout Managers 341
8.13.5 Focus Listener 341
8.13.6 Key Listener 343
8.13.7 Mouse Listener 344
8.13.8 MVC 345
8.14 Exercises 348
Chapter 9 Multithreading 351
9.1 Processes and Threads 351
9.2 Threads in Java 354
9.3 Thread States 357
9.4 Thread Scheduling and Priority 359
9.5 Sharing Access to Data 361
9.6 Synchronized Methods 363
9.6.1 Motivation 364
9.6.2 Stack with Synchronized Methods 367
9.6.3 Producer and Consumer Problem 368
9.7 Atomic Variables 373
9.8 Executors 377
9.9 Callable & Future 380
9.10 BlockingQueue 382
9.11 New Terminology 385
9.12 New Words and Expressions 386
9.13 Hands on Lab 386
9.13.1 Unresponsive User Interface 386
9.13.2 Thread Priority 386
9.13.3 Unsynchronized Counter 387
9.13.4 Volatile 390
9.13.5 Synchronized Methods 390
9.13.6 Atomic Variables 390
9.13.7 Executor 390
9.13.8 Blocking Queue 391
9.14 Exercises 394
Appendix Ⅰ Java Modifiers 396
Appendix Ⅱ Java Documentation 397
Appendix Ⅲ Unicode Chart(Basic Latin) 402
Appendix Ⅳ Helpful Eclipse Shortcuts 406
References 408
- 《市政工程基础》杨岚编著 2009
- 《零基础学会素描》王金著 2019
- 《高级英语阅读与听说教程》刘秀梅编著 2019
- 《计算机网络与通信基础》谢雨飞,田启川编著 2019
- 《生物质甘油共气化制氢基础研究》赵丽霞 2019
- 《看图自学吉他弹唱教程》陈飞编著 2019
- 《激光加工实训技能指导理实一体化教程 下》王秀军,徐永红主编;刘波,刘克生副主编 2017
- 《AutoCAD 2019 循序渐进教程》雷焕平,吴昌松,陈兴奎主编 2019
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《花时间 我的第一堂花艺课 插花基础技法篇》(日)花时间编辑部编;陈洁责编;冯莹莹译 2020
- 《市政工程基础》杨岚编著 2009
- 《家畜百宝 猪、牛、羊、鸡的综合利用》山西省商业厅组织技术处编著 1959
- 《《道德经》200句》崇贤书院编著 2018
- 《高级英语阅读与听说教程》刘秀梅编著 2019
- 《计算机网络与通信基础》谢雨飞,田启川编著 2019
- 《看图自学吉他弹唱教程》陈飞编著 2019
- 《法语词汇认知联想记忆法》刘莲编著 2020
- 《培智学校义务教育实验教科书教师教学用书 生活适应 二年级 上》人民教育出版社,课程教材研究所,特殊教育课程教材研究中心编著 2019
- 《国家社科基金项目申报规范 技巧与案例 第3版 2020》文传浩,夏宇编著 2019
- 《流体力学》张扬军,彭杰,诸葛伟林编著 2019
- 《大学计算机实验指导及习题解答》曹成志,宋长龙 2019
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《大学生心理健康与人生发展》王琳责任编辑;(中国)肖宇 2019
- 《大学英语四级考试全真试题 标准模拟 四级》汪开虎主编 2012
- 《大学英语教学的跨文化交际视角研究与创新发展》许丽云,刘枫,尚利明著 2020
- 《北京生态环境保护》《北京环境保护丛书》编委会编著 2018
- 《复旦大学新闻学院教授学术丛书 新闻实务随想录》刘海贵 2019
- 《大学英语综合教程 1》王佃春,骆敏主编 2015
- 《大学物理简明教程 下 第2版》施卫主编 2020
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019