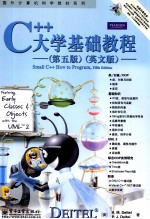
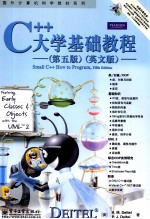
C++大学基础教程 英文版PDF电子书下载
- 电子书积分:17 积分如何计算积分?
- 作 者:H·M·Deitel著
- 出 版 社:北京:电子工业出版社
- 出版年份:2010
- ISBN:9787121118586
- 页数:573 页
Chapter 1 Introduction to Computers,the Internet and World Wide Web 1
1.1 Introduction 2
1.2 What Is a Computer? 3
1.3 Computer Organization 3
1.4 Early Operating Systens 4
1.5 Personal,Distributed and Client/Server Computing 4
1.6 The Internet and the World Wide Web 4
1.7 Machine Languages,Assembly Languages and High-Level Languages 5
1.8 History of C and C++ 6
1.9 C++Standard Library 6
1.10 History of Java 7
1.11 FORTRAN,COBOL,Pascal and Ada 7
1.12 Basic,Visual Basic,Visual C++,C# and .NET 8
1.13 Key Software Trend:Object Technology 8
1.14 Typical C++Development Environment 9
1.15 Notes About C++and Small C++How to Program,5/e 11
1.16 Test-Driving a C++Application 12
1.17 Introduction to Object Technology and the UML 16
1.18 Wrap-Up 20
1.19 Web Resources 21
Chapter 2 Introduction to C++Programming 28
2.1 Introduction 28
2.2 First Program in C++:Printing a Line of Text 29
2.3 Modifying Our First C++Progran 31
2.4 Another C++Program:Adding Integers 32
2.5 Memory Concepts 35
2.6 Arithmetic 36
2.7 Decision Making:Equality and Relational Operators 39
2.8 Wrap-Up 42
Chapter 3 Introduction to Classes and Objects 50
3.1 Introduction 51
3.2 Classes,Objects,Member Functions and Data Members 51
3.3 Overview of the Chapter Examples 52
3.4 Defining a Class with a Member Function 52
3.5 Defining a Member Function with a Parameter 55
3.6 Data Members,set Functions and get Functions 57
3.7 Initializing Objects with Constructors 62
3.8 Placing a Class in a Separate File for Reusability 65
3.9 Separating Interface from Implementation 68
3.10 Validating Data with set Functions 72
3.11 Wrap-Up 76
Chapter 4 Control Statements:Part 1 82
4.1 Introduction 83
4.2 Algorithms 83
4.3 Pseudocode 83
4.4 Control Structures 84
4.5 if Selection Statement 87
4.6 if...else Double-Selection Statement 88
4.7 while Repetition Statement 92
4.8 Formulating Algorithms:Counter-Controlled Repetition 93
4.9 Formulating Algorithms:Sentinel-Controlled Repetition 97
4.10 Formulating Algorithms:Nested Control Statements 105
4.11 Assignment Operators 108
4.12 Increment and Decrement Operators 109
4.13 Wrap-Up 111
Chapter 5 Control Statements:Part 2 124
5.1 Introduction 125
5.2 Essentials ofCounter-Controlled Repetition 125
5.3 for Repetition Statement 126
5.4 Examples Using the for Statement 130
5.5 do...while Repetition Statement 133
56 swi tch Multiple-Selection Statement 134
5.7 break and continue Statements 141
5.8 Logical Operators 143
5.9 Confusing Equality(==)and Assignment(=)Operators 146
5.10 Structured Programming Summary 147
5.11 Wrap-Up 150
Chapter 6 Functions and an Introduction to Recursion 159
6.1 Introduction 160
6.2 Program Components in C++ 160
6.3 Math Library Functions 162
6.4 Function Deftnitions with Multiple Parameters 162
6.5 Function Prototypes and Argument Coercion 166
6.6 C++Standard Library Header Files 168
6.7 Case Study:Random Number Generation 169
6.8 Case Study:Garne ofChance and Introducing enum 173
6.9 Storage Classes 175
6.10 Scope Rules 177
6.11 Function Call Stack andActivation Records 180
6.12 Functions with Empty Parameter Lists 182
6.13 Inline Functions 183
6.14 References and Reference Parameters 184
6.15 DefaultArguments 188
6.16 Unary Scope Resolution Operator 189
6.17 Function Overloading 190
6.18 Function Templates 192
6.19 Recursion 194
6.20 Example Using Recursion:Fibonacci Series 196
6.21 Recursion vs.Iteration 199
6.22 Wrap-Up 200
Chapter 7 Arrays and Vectors 218
7.1 Introduction 218
7.2 Arravs 219
7.3 Declaring Arrays 220
7.4 Examples Using Arrays 221
7.5 Passing Arrays to Functions 232
7.6 Case Study:Class GradeBook Using an Array to Store Grades 235
7.7 Searching Arrays with Linear Search 240
7.8 Sorting Arrays with Insertion Sort 241
7.9 Multidimensional Arrays 243
7.10 Case Study:Class GradeBook Using a Two-Dimensional Array 245
7.11 Introduction to C++Standard Library Class Template vector 250
7.12 Wrap-Up 253
Chapter 8 Pointers and Pointer-Based Strings 267
8.1 Introduction 268
8.2 Pointer Variable Declarations and Initialization 268
8.3 Pointer Operators 269
8.4 Passing Arguments to Functions by Reference with Pointers 271
8.5 Using const with Pointers 274
8.6 Selection Sort Using Pass-by-Reference 279
8.7 sizeof Operators 281
8.8 Pointer Expressions and Pointer Arithmetic 283
8.9 Relationship Between Pointers and Arrays 285
8.10 Arrays of Pointers 288
8.11 Case Study:Card Shuffling and Dealing Simulation 289
8.12 Function Pointers 293
8.13 Introduction to Pointer-Based String Processing 297
8.14 Wrap-Up 304
Chapter 9 Classes:A Deeper Look,Part 1 324
9.1 Introduction 325
9.2 Time Class Case Study 325
9.3 Class Scope and Accessing Class Members 329
9.4 Separating Interface from Implementation 331
9.5 Access Functions and Utility Functions 332
9.6 Time Class Case Study:Constructors with Default Arguments 334
9.7 Destructors 337
9.8 When Constructors and Destructors Are Called 338
9.9 Time Class Case Study:A Subtle Trap—Returning a Reference to a private Data Member 340
9.10 Default Memberwise Assignment 342
9.11 Software Reusability 344
9.12 Wrap-Up 344
Chapter 10 Classes:A Deeper Look,Part 2 350
10.1 Introduction 351
10.2 const(Constant)Objects and const Member Functions 351
10.3 Composition:Objects as Members of Classes 357
10.4 friend Functions and friend Classes 362
10.5 Using the this Pointer 365
10.6 Dynamic Memory Management with Operators new and delete 368
10.7 static Class Members 370
10.8 Data Abstraction and Information Hiding 374
10.9 Container Classes and Iterators 376
10.10 Proxy Classes 376
10.11 Wrap-Up 379
Chapter 11 Operator Overloading;String and Array Objects 384
11.1 Introduction 385
11.2 Fundamentals of Operator Overloading 385
11.3 Restrictions on Operator Overloading 386
11.4 OperatorFunctions as Class Members vs.Global Functions 387
11.5 Overloading Stream Insertion and Stream Extraction Operators 388
11.6 Overloading Unary Operators 391
11.7 Overloading Binary Operators 391
11.8 Case Study:Array Class 392
11.9 Converting between Types 400
11.10 Case Study:String Class 401
11.11 Overloading++and-- 410
11.12 Case Study:A Date Class 411
11.13 Standard Library Class string 414
11.14 explicit Constructors 417
11.15 Wrap-Up 419
Chapter 12 Object-Oriented Programming:Inheritance 428
12.1 Introduction 429
12.2 Base Classes and Derived Classes 430
12.3 protected Members 431
12.4 Relationship between Base Classes and Derived Classes 432
12.5 Constructors and Destructors in Derived Classes 453
12.6 public,protected and private Inheritance 459
12.7 Software Engineering with Inheritance 459
12.8 Wrap-Up 460
Chapter 13 Object-Oriented Programming:Polymorphism 465
13.1 Introduction 466
13.2 Polymorphism Examples 467
13.3 Relationships Among Objects in an Inheritance Hierarchy 468
13.4 Type Fields and switch Statements 480
13.5 Abstract Classes and Pure virtual Functions 480
13.6 Case Study:Payroll System Using Polymorphism 482
13.7 (Optional)Polymorphism,Virtual Functions and Dynamic Binding"Under the Hood" 494
13.8 Case Study:Payroll System Using Polymorphism and Run-Time Type Information with Downcasting,dynamic_cast,typeid and type_info 497
13.9 Virtual Destructors 499
13.10 Wrap-Up 500
Appendix A Operator Precedence and Associativity Chart 504
Appendix B ASCII Character Set 506
Appendix C Fundamental Types 507
Appendix D Number Systems 508
Appendix E C++Internet and Web Resources 518
Appendix F Using the Visual Studio.NET Debugger 522
Appendix G Using the GNU C++Debugger 534
Bibliography 547
Index 551
- 《市政工程基础》杨岚编著 2009
- 《零基础学会素描》王金著 2019
- 《高级英语阅读与听说教程》刘秀梅编著 2019
- 《计算机网络与通信基础》谢雨飞,田启川编著 2019
- 《大学计算机实验指导及习题解答》曹成志,宋长龙 2019
- 《生物质甘油共气化制氢基础研究》赵丽霞 2019
- 《看图自学吉他弹唱教程》陈飞编著 2019
- 《激光加工实训技能指导理实一体化教程 下》王秀军,徐永红主编;刘波,刘克生副主编 2017
- 《AutoCAD 2019 循序渐进教程》雷焕平,吴昌松,陈兴奎主编 2019
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《中风偏瘫 脑萎缩 痴呆 最新治疗原则与方法》孙作东著 2004
- 《水面舰艇编队作战运筹分析》谭安胜著 2009
- 《王蒙文集 新版 35 评点《红楼梦》 上》王蒙著 2020
- 《TED说话的力量 世界优秀演讲者的口才秘诀》(坦桑)阿卡什·P.卡里亚著 2019
- 《燕堂夜话》蒋忠和著 2019
- 《经久》静水边著 2019
- 《魔法销售台词》(美)埃尔默·惠勒著 2019
- 《微表情密码》(波)卡西亚·韦佐夫斯基,(波)帕特里克·韦佐夫斯基著 2019
- 《看书琐记与作文秘诀》鲁迅著 2019
- 《酒国》莫言著 2019
- 《电子测量与仪器》人力资源和社会保障部教材办公室组织编写 2009
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《北京生态环境保护》《北京环境保护丛书》编委会编著 2018
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019
- 《通信电子电路原理及仿真设计》叶建芳 2019
- 《高等院校旅游专业系列教材 旅游企业岗位培训系列教材 新编北京导游英语》杨昆,鄢莉,谭明华 2019
- 《电子应用技术项目教程 第3版》王彰云 2019
- 《中国十大出版家》王震,贺越明著 1991
- 《近代民营出版机构的英语函授教育 以“商务、中华、开明”函授学校为个案 1915年-1946年版》丁伟 2017