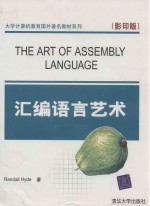
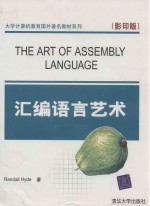
汇编语言艺术 影印版PDF电子书下载
- 电子书积分:23 积分如何计算积分?
- 作 者:RandallHyde著
- 出 版 社:北京:清华大学出版社
- 出版年份:2005
- ISBN:7302104352
- 页数:891 页
1 HELLO,WORLD OF ASSEMBLY LANGUAGE 1
1.1 Chapter Overview 1
1.2 The Anatomy of an HLA Program 2
1.3 Running Your First HLA Program 4
1.4 Some Basic HLA Data Declarations 5
1.5 Boolean Values 7
1.6 Character Values 8
1.7 An Introduction to the Intel 80x86 CPU Family 8
1.7.1 The Memory Subsystem 11
1.8 Some Basic Machine Instructions 14
1.9 Some Basic HLA Control Structures 18
1.9.1 Boolean Expressions in HLA Statements 18
1.9.2 The HLA IF..THEN..ELSEIF..ELSE..ENDIF Statement 20
1.9.3 Conjunction,Disjunction,and Negation in Boolean Expressions 22
1.9.4 The WHILE..ENDWHILE Statement 25
1.9.5 The FOR..ENDFOR Statement 25
1.9.6 The REPEAT..UNTILStatement 26
1.9.7 The BREAK and BREAKIF Statements 27
1.9.8 The FOREVER..ENDFOR Statement 28
1.9.9 The TRY..EXCEPT1ON..ENDTRYStatement 28
1.10 Introduction to the HLA Standard Library 32
1.10.1 Predefined Constants in the STDIO Module 33
1.10.2 Standard In and Standard Out 34
1.10.3 The stdout.newln Routine 34
1.10.4 The stdout.putiX Routines 34
1.10.5 The stdout.putiXSize Routines 35
1.10.6 The stdout.put Routine 36
1.10.7 The stdin.getc Routine 38
1.10.8 The stdin.getiX Routines 39
1.10.9 The stdin.readLn and stdin.flushlnput Routines 40
1.10.10 The stdin.get Routine 41
1.11 Additional Details About TRY..ENDTRY 42
1.11.1 Nesting TRY..ENDTRY Statements 43
1.11.2 The UNPROTECTED Clause in a TRY..ENDTRY Statement 45
1.11.3 The ANYEXCEPTION Clause in a TRY..ENDTRY Statement 48
1.11.4 Registers and the TRY..ENDTRY Statement 48
1.12 High Level Assembly Language vs.Low Level Assembly 50
1.13 For More Information 51
2 DATA REPRESENTATION 53
2.1 Chapter Overview 53
2.2 Numbering Systems 54
2.2.1 A Review of the Decimal System 54
2.2.2 The Binary Numbering System 54
2.2.3 Binary Formats 56
2.3 The Hexadecimal Numbering System 57
2.4 Data Organization 59
2.4.1 Bits 59
2.4.2 Nibbles 60
2.4.3 Bytes 61
2.4.4 Words 62
2.4.5 Double Words 63
2.4.6 Quad Words and Long Words 64
2.5 Arithmetic Operations on Binary and Hexadecimal Numbers 65
2.6 A Note About Numbers vs.Representation 66
2.7 Logical Operations on Bits 69
2.8 Logical Operations on Binary Numbers and Bit Strings 71
2.9 Signed and Unsigned Numbers 73
2.10 Sign Extension,Zero Extension,Contraction,and Saturation 78
2.11 Shifts and Rotates 82
2.12 Bit Fields and Packed Data 87
2.13 An Introduction to Floating Point Arithmetic 92
2.13.1 IEEE Floating Point Formats 95
2.13.2 HLA Support for Floating Point Values 99
2.14 Binary Coded Decimal(BCD)Representation 102
2.15 Characters 104
2.15.1 The ASCII Character Encoding 104
2.15.2 HLA Support for ASCII Characters 108
2.16 The Unicode Character Set 112
2.17 For More Information 113
3 MEMORY ACCESS AND ORGANIZATION 115
3.1 Chapter Overview 115
3.2 The 80x86 Addressing Modes 115
3.2.1 80x86 Register Addressing Modes 116
3.2.2 80x86 32-Bit Memory Addressing Modes 117
3.3 Run-Time Memory Organization 124
3.3.1 The Code Section 125
3.3.2 The Static Sections 127
3.3.3 The Read-Only Data Section 128
3.3.4 The Storage Section 129
3.3.5 The @NOSTORAGE Attribute 129
3.3.6 The Var Section 130
3.3.7 Organization of Declaration Sections Within Your Programs 131
3.4 How HLA Allocates Memory for Variables 132
3.5 HLA Support for Data Alignment 133
3.6 Address Expressions 136
3.7 Type Coercion 139
3.8 Register Type Coercion 141
3.9 The Stack Segment and the PUSH and POP Instructions 142
3.9.1 The Basic PUSH Instruction 142
3.9.2 The Basic POP Instruction 144
3.9.3 Preserving Registers with the PUSH and POP Instructions 146
3.9.4 The Stack Is a LIFO Data Structure 146
3.9.5 Other PUSH and POP Instructions 149
3.9.6 Removing Data from the Stack Without Popping It 150
3.9.7 Accessing Data You've Pushed on the Stack Without Popping It 153
3.10 Dynamic Memory Allocation and the Heap Segment 154
3.11 The INC and DEC Instructions 159
3.12 Obtaining the Address of a Memory Object 159
3.13 For More Information 160
4 CONSTANTS,VARIABLES,AND DATA TYPES 160
4.1 Chapter Overview 161
4.2 Some Additional Instructions:INTMUL,BOUND,INTO 162
4.3 The TBYTE Data Types 166
4.4 HLA Constant and Value Declarations 167
4.4.1 Constant Types 170
4.4.2 String and Character Literal Constants 171
4.4.3 String and Text Constants in the CONST Section 174
4.4.4 Constant Expressions 175
4.4.5 Multiple CONST Sections and Their Order in an HLA Program 178
4.4.6 The HLA VAL Section 178
4.4.7 Modifying VAL Objects at Arbitrary Points in Your Programs 179
4.5 The HLA TYPE Section 180
4.6 ENUM and HLA Enumerated Data Types 181
4.7 Pointer Data Types 182
4.7.1 Using Pointers in Assembly Language 184
4.7.2 Declaring Pointers in HLA 185
4.7.3 Pointer Constants and Pointer Constant Expressions 185
4.7.4 Pointer Variables and Dynamic Memory Allocation 187
4.7.5 Common Pointer Problems 188
4.8 The HLA Standard Library CHARS.HHF Module 192
4.9 Composite Data Types 195
4.10 Character Strings 195
4.11 HLA Strings 198
4.12 Accessing the Characters Within a String 204
4.13 The HLA String Module and Other String-Related Routines 206
4.14 In-Memory Conversions 219
4.15 Character Sets 220
4.16 Character Set Implementation in HLA 221
4.17 HLA Character Set Constants and Character Set Expressions 223
4.18 The IN Operator in HLA HLL Boolean Expressions 224
4.19 Character Set Support in the HLA Standard Library 225
4.20 Using Character Sets in Your HLA Programs 229
4.21 Arrays 230
4.22 Declaring Arrays in Your HLA Programs 231
4.23 HLA Array Constants 232
4.24 Accessing Elements of a Single Dimension Array 233
4.24.1 Sorting an Array of Values 235
4.25 Multidimensional Arrays 237
4.25.1 Row Major Ordering 238
4.25.2 Column Major Ordering 242
4.26 Allocating Storage for Multidimensional Arrays 243
4.27 Accessing Multidimensional Array Elements in Assembly Language 245
4.28 Large Arrays and MASM(Windows Programmers Only) 246
4.29 Records 247
4.30 Record Constants 249
4.31 Arrays of Records 250
4.32 Arrays/Records as Record Fields 251
4.33 Controlling Field Offsets Within a Record 255
4.34 Aligning Fields Within a Record 256
4.35 Pointers to Records 257
4.36 Unions 259
4.37 Anonymous Unions 262
4.38 Variant Types 262
4.39 Union Constants 263
4.40 Namespaces 264
4.41 Dynamic Arrays in Assembly Language 268
4.42 HLA Standard Library Array Support 270
4.43 For More Information 273
5 PROCEDURES AND UNITS 275
5.1 Chapter Overview 275
5.2 Procedures 276
5.3 Saving the State of the Machine 278
5.4 Prematurely Returning from a Procedure 282
5.5 Local Variables 283
5.6 Other Local and Global Symbol Types 289
5.7 Parameters 289
5.7.1 Pass by Value 290
5.7.2 Pass by Reference 293
5.8 Functions and Function Results 296
5.8.1 Returning Function Results 297
5.8.2 Instruction Composition in HLA 298
5.8.3 The HLA @RETURNS Option in Procedures 301
5.9 Recursion 303
5.10 Forward Procedures 307
5.11 Low Level Procedures and the CALL Instruction 308
5.12 Procedures and the Stack 311
5.13 Activation Records 314
5.14 The Standard Entry Sequence 317
5.15 The Standard Exit Sequence 318
5.16 Low Level Implementation of Automatic(Local)Variables 320
5.17 Low Level Parameter Implementation 322
5.17.1 Passing Parameters in Registers 322
5.17.2 Passing Parameters in the Code Stream 325
5.17.3 Passing Parameters on the Stack 328
5.18 Procedure Pointers 350
5.19 Procedure Parameters 354
5.20 Untyped Reference Parameters 355
5.21 Managing Large Programs 356
5.22 The #INCLUDE Directive 357
5.23 Ignoring Duplicate #INCLUDE Operations 358
5.24 UNITs and the EXTERNAL Directive 359
5.24.1 Behavior of the EXTERNAL Directive 364
5.24.2 Header Files in HLA 365
5.25 Namespace Pollution 366
5.26 For More Information 369
6 ARITHMETIC 371
6.1 Chapter Overview 371
6.2 80x86 Integer Arithmetic Instructions 371
6.2.1 The MUL and IMUL Instructions 371
6.2.2 The DIV and IDIV Instructions 375
6.2.3 The CMP Instruction 378
6.2.4 The SETcc Instructions 382
6.2.5 The TEST Instruction 384
6.3 Arithmetic Expressions 385
6.3.1 Simple Assignments 386
6.3.2 Simple Expressions 387
6.3.3 Complex Expressions 389
6.3.4 Commutative Operators 395
6.4 Logical(Boolean)Expressions 396
6.5 Machine and Arithmetic Idioms 398
6.5.1 Multiplying Without MUL,IMUL,or INTMUL 398
6.5.2 Division Without DIV or IDIV 400
6.5.3 Implementing Modulo-N Counters with AND 400
6.5.4 Careless Use of Machine Idioms 401
6.6 Floating Point Arithmetic 401
6.6.1 FPU Registers 402
6.6.2 FPU Data Types 408
6.6.3 The FPU Instruction Set 410
6.6.4 FPU Data Movement Instructions 410
6.6.5 Conversions 412
6.6.6 Arithmetic Instructions 414
6.6.7 Comparison Instructions 420
6.6.8 Constant Instructions 422
6.6.9 Transcendental Instructions 422
6.6.10 Miscellaneous Instructions 424
6.6.11 Integer Operations 426
6.7 Converting Floating Point Expressions to Assembly Language 426
6.7.1 Converting Arithmetic Expressions to Postfix Notation 428
6.7.2 Converting Postfix Notation to Assembly Language 430
6.8 HLA Standard Library Support for Floating Point Arithmetic 431
6.8.1 The stdin.getf and fileio.getf Functions 431
6.8.2 Trigonometric Functions in the HLA Math Library 432
6.8.3 Exponential and Logarithmic Functions in the HLA Math Library 433
6.9 Putting It All Together 434
7 LOW LEVEL CONTROL STRUCTURES 434
7.1 Chapter Overview 435
7.2 Low Level Control Structures 435
7.3 Statement Labels 436
7.4 Unconditional Transfer of Control(JMP) 438
7.5 The Conditional Jump Instructions 441
7.6 "Medium Level" Control Structures:JT and JF 444
7.7 Implementing Common Control Structures in Assembly Language 445
7.8 Introduction to Decisions 445
7.8.1 IF..THEN..ELSE Sequences 447
7.8.2 Translating HLA IF Statements into Pure Assembly Language 451
7.8.3 Implementing Complex IF Statements Using Complete Boolean Evaluation 456
7.8.4 Short-Circuit Boolean Evaluation 457
7.8.5 Short-Circuit vs.Complete Boolean Evaluation 459
7.8.6 Efficient Implementation of IF Statements in Assembly Language 461
7.8.7 SWITCH/CASE Statements 466
7.9 State Machines and Indirect Jumps 477
7.10 Spaghetti Code 480
7.11 Loops 481
7.11.1 WHILE Loops 482
7.11.2 REPEAT..UNTILLoops 483
7.11.3 FOREVER..ENDFOR Loops 484
7.11.4 FOR Loops 485
7.11.5 The BREAK and CONTINUE Statements 486
7.11.6 Register Usage and Loops 490
7.12 Performance Improvements 491
7.12.1 Moving the Termination Condition to the End of a Loop 492
7.12.2 Executing the Loop Backward 494
7.12.3 Loop Invariant Computations 495
7.12.4 Unraveling Loops 496
7.12.5 Induction Variables 498
7.13 Hybrid Control Structures in HLA 499
7.14 For More Information 501
8 FILES 503
8.1 Chapter Overview 503
8.2 File Organization 503
8.2.1 Files as Lists of Records 504
8.2.2 Binary vs.Text Files 506
8.3 Sequential Files 508
8.4 Random Access Files 516
8.5 ISAM(Indexed Sequential Access Method)Files 520
8.6 Truncating a File 524
8.7 For More Information 525
9 ADVANCED ARITHMETIC 527
9.1 Chapter Overview 527
9.2 Multiprecision Operations 528
9.2.1 HLA Standard Library Support for Extended Precision Operations 528
9.2.2 Multiprecision Addition Operations 531
9.2.3 Multiprecision Subtraction Operations 534
9.2.4 Extended Precision Comparisons 535
9.2.5 Extended Precision Multiplication 539
9.2.6 Extended Precision Division 543
9.2.7 Extended Precision NEG Operations 553
9.2.8 Extended Precision AND Operations 555
9.2.9 Extended Precision OR Operations 555
9.2.10 Extended Precision XOR Operations 556
9.2.11 Extended Precision NOT Operations 556
9.2.12 Extended Precision Shift Operations 556
9.2.13 Extended Precision Rotate Operations 560
9.2.14 Extended Precision I/O 561
9.3 Operating on Different-Sized Operands 582
9.4 Decimal Arithmetic 584
9.4.1 Literal BCD Constants 585
9.4.2 The 80x86 DAA and DAS Instructions 586
9.4.3 The 80x86 AAA,AAS,AAM,and AAD Instructions 588
9.4.4 Packed Decimal Arithmetic Using the FPU 589
9.5 Tables 591
9.5.1 Function Computation via Table Look-Up 592
9.5.2 Domain Conditioning 597
9.5.3 Generating Tables 598
9.5.4 Table Look-Up Performance 601
9.6 For More Information 602
10 MACROS AND THE HLA COMPILE TIME LANGUAGE 602
10.1 Chapter Overview 603
10.2 Introduction to the Compile Time Language(CTL) 603
10.3 The #PRINT and #ERROR Statements 605
10.4 Compile Time Constants and Variables 607
10.5 Compile Time Expressions and Operators 607
10.6 Compile Time Functions 610
10.6.1 Type Conversion Compile Time Functions 611
10.6.2 Numeric Compile Time Functions 612
10.6.3 Character Classification Compile Time Functions 613
10.6.4 Compile Time String Functions 613
10.6.5 Compile Time Pattern Matching Functions 614
10.6.6 Compile Time Symbol Information 615
10.6.7 Miscellaneous Compile Time Functions 616
10.6.8 Compile Time Type Conversions of TEXT Objects 617
10.7 Conditional Compilation(Compile Time Decisions) 618
10.8 Repetitive Compilation(Compile Time Loops) 623
10.9 Macros(Compile Time Procedures) 627
10.9.1 Standard Macros 627
10.9.2 Macro Parameters 629
10.9.3 Local Symbols in a Macro 636
10.9.4 Macros as Compile Time Procedures 639
10.9.5 Simulating Function Overloading with Macros 640
10.10 Writing Compile Time "Programs" 646
10.10.1 Constructing Data Tables at Compile Time 646
10.10.2 Unrolling Loops 651
10.11 Using Macros in Different Source Files 653
10.12 For More Information 653
11 BIT MANIPULATION 655
11.1 Chapter Overview 655
11.2 What Is Bit Data,Anyway? 656
11.3 Instructions That Manipulate Bits 657
11.4 The Carry Flag as a Bit Accumulator 665
11.5 Packing and Unpacking Bit Strings 666
11.6 Coalescing Bit Sets and Distributing Bit Strings 669
11.7 Packed Arrays of Bit Strings 671
11.8 Searching for a Bit 673
11.9 Counting Bits 676
11.10 Reversing a Bit String 679
11.11 Merging Bit Strings 681
11.12 Extracting Bit Strings 682
11.13 Searching for a Bit Pattern 683
11.14 The HLA Standard Library Bits Module 684
11.15 For More Information 687
12 THE STRING INSTRUCTIONS 687
12.1 Chapter Overview 689
12.2 The 80x86 String Instructions 690
12.2.1 How the String Instructions Operate 690
12.2.2 The REP/REPE/REPZ and REPNZ/REPNE Prefixes 691
12.2.3 The Direction Flag 692
12.2.4 The MOVS Instruction 694
12.2.5 The CMPS Instruction 700
12.2.6 The SCAS Instruction 703
12.2.7 The STOS Instruction 704
12.2.8 The LODS Instruction 705
12.2.9 Building Complex String Functions from LODS and STOS 705
12.3 Performance of the 80x86 String Instructions 706
12.4 For More Information 707
13 THE MMX INSTRUCTION SET 709
13.1 Chapter Overview 709
13.2 Determining Whether a CPU Supports the MMX Instruction Set 710
13.3 The MMX Programming Environment 711
13.3.1 The MMX Registers 711
13.3.2 The MMX Data Types 713
13.4 The Purpose of the MMX Instruction Set 714
13.5 Saturation Arithmetic and Wrap-Around Mode 714
13.6 MMX Instruction Operands 715
13.7 MMX Technology Instructions 717
13.7.1 MMX Data Transfer Instructions 718
13.7.2 MMX Conversion Instructions 718
13.7.3 MMX Packed Arithmetic Instructions 723
13.7.4 MMX Logical Instructions 726
13.7.5 MMX Comparison Instructions 727
13.7.6 MMX Shift Instructions 731
13.7.7 The EMMS Instruction 733
13.8 The MMX Programming Paradigm 734
13.9 For More Information 745
14 CLASSES AND OBJECTS 747
14.1 Chapter Overview 747
14.2 General Principles 748
14.3 Classes in HLA 750
14.4 Objects 753
14.5 Inheritance 755
14.6 Overriding 756
14.7 Virtual Methods vs.Static Procedures 757
14.8 Writing Class Methods and Procedures 759
14.9 Object Implementation 764
14.9.1 Virtual Method Tables 767
14.9.2 Object Representation with Inheritance 769
14.10 Constructors and Object Initialization 773
14.10.1 Dynamic Object Allocation Within the Constructor 775
14.10.2 Constructors and Inheritance 777
14.10.3 Constructor Parameters and Procedure Overloading 781
14.11 Destructors 782
14.12 HLA's "_initialize_" and "_finalize_" Strings 783
14.13 Abstract Methods 789
14.14 Run-Time Type Information(RTTI) 792
14.15 Calling Base Class Methods 794
14.16 For More Information 795
15 MIXED LANGUAGE PROGRAMMING 797
15.1 Chapter Overview 797
15.2 Mixing HLA and MASM/Gas Code in the Same Program 798
15.2.1 In-Line(MASM/Gas)Assembly Code in Your HLA Programs 798
15.2.2 Linking MASM/Gas-Assembled Modules with HLA Modules 801
15.3 Programming in Delphi/Kylix and HLA 805
15.3.1 Linking HLA Modules with Delphi/Kylix Programs 806
15.3.2 Register Preservation 810
15.3.3 Function Results 811
15.3.4 Calling Conventions 817
15.3.5 Pass by Value,Reference,CONST,and OUT in Kylix 823
15.3.6 Scalar Data Type Correspondence Between Delphi/Kylix and HLA 825
15.3.7 Passing String Data Between Delphi/Kylix and HLA Code 826
15.3.8 Passing Record Data Between HLA and Kylix 829
15.3.9 Passing Set Data Between Delphi/Kylix and HLA 833
15.3.10 Passing Array Data Between HLA and Delphi/Kylix 834
15.3.11 Referencing Delphi/Kylix Objects from HLA Code 834
15.4 Programming in C/C++ and HLA 837
15.4.1 Linking HLA Modules with C/C++ Programs 839
15.4.2 Register Preservation 842
15.4.3 Function Results 842
15.4.4 Calling Conventions 842
15.4.5 Pass by Value and Reference in C/C++ 847
15.4.6 Scalar Data Type Correspondence Between C/C++ and HLA 847
15.4.7 Passing String Data Between C/C++ and HLA Code 849
15.4.8 Passing Record/Structure Data Between HLA and C/C++ 849
15.4.9 Passing Array Data Between HLA and C/C++ 851
15.5 For More Information 852
A ASCII CHARACTER SET 853
B THE 80X86 INSTRUCTION SET 857
INDEX 889
- 《东北民歌文化研究及艺术探析》(中国)杨清波 2019
- 《莼江曲谱 2 中国昆曲博物馆藏稀见昆剧手抄曲谱汇编之一》郭腊梅主编;孙伊婷副主编;孙文明,孙伊婷编委;中国昆曲博物馆编 2018
- 《舞剧艺术论》张麟著 2019
- 《中国陈设艺术史》赵囡囡著 2019
- 《莼江曲谱 1 中国昆曲博物馆藏稀见昆剧手抄曲谱汇编之一》郭腊梅主编;孙伊婷副主编;孙文明,孙伊婷编委;中国昆曲博物馆编 2018
- 《全国校外艺术课堂新形态示范教材系列 少儿钢琴表演曲集》唐冠祥编著 2019
- 《景观艺术设计》林春水,马俊 2019
- 《近代体育游戏教育史料汇编 第1辑 1》王强主编 2016
- 《韩愈散文艺术论》孙昌武著 2018
- 《程序逻辑及C语言编程》卢卫中,杨丽芳主编 2019
- 《中风偏瘫 脑萎缩 痴呆 最新治疗原则与方法》孙作东著 2004
- 《水面舰艇编队作战运筹分析》谭安胜著 2009
- 《王蒙文集 新版 35 评点《红楼梦》 上》王蒙著 2020
- 《TED说话的力量 世界优秀演讲者的口才秘诀》(坦桑)阿卡什·P.卡里亚著 2019
- 《燕堂夜话》蒋忠和著 2019
- 《经久》静水边著 2019
- 《魔法销售台词》(美)埃尔默·惠勒著 2019
- 《微表情密码》(波)卡西亚·韦佐夫斯基,(波)帕特里克·韦佐夫斯基著 2019
- 《看书琐记与作文秘诀》鲁迅著 2019
- 《酒国》莫言著 2019
- 《大学计算机实验指导及习题解答》曹成志,宋长龙 2019
- 《指向核心素养 北京十一学校名师教学设计 英语 七年级 上 配人教版》周志英总主编 2019
- 《大学生心理健康与人生发展》王琳责任编辑;(中国)肖宇 2019
- 《大学英语四级考试全真试题 标准模拟 四级》汪开虎主编 2012
- 《大学英语教学的跨文化交际视角研究与创新发展》许丽云,刘枫,尚利明著 2020
- 《北京生态环境保护》《北京环境保护丛书》编委会编著 2018
- 《复旦大学新闻学院教授学术丛书 新闻实务随想录》刘海贵 2019
- 《大学英语综合教程 1》王佃春,骆敏主编 2015
- 《大学物理简明教程 下 第2版》施卫主编 2020
- 《指向核心素养 北京十一学校名师教学设计 英语 九年级 上 配人教版》周志英总主编 2019