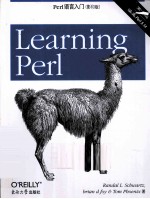
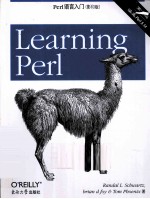
Perl语言入门 原书第6版PDF电子书下载
- 电子书积分:13 积分如何计算积分?
- 作 者:RandalL.Schwartz,TomPhoenixBrianDFoy著
- 出 版 社:南京:东南大学出版社
- 出版年份:2011
- ISBN:9787564130848
- 页数:368 页
1.Introduction 1
Questions and Answers 1
Is This the Right Book for You? 1
Why Are There So Many Footnotes? 2
What About the Exercises and Their Answers? 3
What Do Those Numbers Mean at the Start of the Exercise? 4
What If I'm a Perl Course Instructor? 4
What Does"Perl"Stand For? 4
Why Did Larry Create Perl? 5
Why Didn't Larry Just Use Some Other Language? 5
Is Perl Easy or Hard? 6
How Did Perl Get to Be So Popular? 7
What's Happening with Perl Now? 7
What's Perl Really Good For? 8
What Is Perl Not Good For? 8
How Can I Get Perl? 9
What Is CPAN? 10
How Can I Get Support for Perl? 10
Are There Any Other Kinds of Support? 10
What If I Find a Bug in Perl? 12
How Do I Make a Perl Program? 12
A Simple Program 13
What's Inside That Program? 15
How Do I Compile My Perl Program? 16
A Whirlwind Tour of Perl 17
Exercises 18
2.Scalar Data 21
Numbers 21
All Numbers Have the Same Format Internally 22
Floating-Point Literals 22
Integer Literals 22
Nondecimal Integer Literals 23
Numeric Operators 23
Strings 24
Single-Quoted String Literals 25
Double-Quoted String Literals 25
String Operators 26
Automatic Conversion Between Numbers and Strings 27
Perl's Built-in Warnings 28
Scalar Variables 29
Choosing Good Variable Names 30
Scalar Assignment 31
Binary Assignment Operators 31
Output with print 32
Interpolation of Scalar Variables into Strings 32
Creating Characters by Code Point 34
Operator Precedence and Associativity 34
Comparison Operators 36
The if Control Structure 37
Boolean Values 38
Getting User Input 39
The chomp Operator 39
The while Control Structure 40
The undef Value 41
The defined Function 42
Exercises 42
3.Lists and Arrays 43
Accessing Elements of an Array 44
Special Array Indices 45
List Literals 46
The qw Shortcut 46
List Assignment 48
The pop and push Operators 49
The shift and unshift Operators 50
The splice Operator 50
Interpolating Arrays into Strings 51
The foreach Control Structure 53
Perl's Favorite Default:$_ 54
The reverse Operator 54
The sort Operator 54
The each Operator 55
Scalar and List Context 55
Using List-Producing Expressions in Scalar Context 57
Using Scalar-Producing Expressions in List Context 58
Forcing Scalar Context 59
<STDIN>in List Context 59
Exercises 60
4.Subroutines 63
Defining a Subroutine 63
Invoking a Subroutine 64
Return Values 64
Arguments 66
Private Variables in Subroutines 68
Variable-Length Parameter Lists 69
A Better&max Routine 69
Empty Parameter Lists 70
Notes on Lexical(my)Variables 71
The use strict Pragma 72
The return Operator 74
Omitting the Ampersand 74
Non-Scalar Return Values 76
Persistent,Private Variables 76
Exercises 78
5.Input and Output 81
Input from Standard Input 81
Input from the Diamond Operator 83
The Invocation Arguments 85
Output to Standard Output 86
Formatted Output with printf 89
Arrays and printf 90
Filehandles 91
Opening a Filehandle 93
Binmoding Filehandles 95
Bad Filehandles 96
Closing a Filehandle 96
Fatal Errors with die 97
Warning Messages with warn 99
Automatically die-ing 99
Using Filehandles 100
Changing the Default Output Filehandle 100
Reopening a Standard Filehandle 101
Output with say 102
Filehandles in a Scalar 103
Exercises 104
6.Hashes 107
What Is a Hash? 107
Why Use a Hash? 109
Hash Element Access 110
The Hash As a Whole 112
Hash Assignment 113
The Big Arrow 114
Hash Functions 115
The keys and values Functions 115
The each Function 116
Typical Use of a Hash 118
The exists Function 118
The delete Function 118
Hash Element Interpolation 119
The %ENV hash 119
Exercises 120
7.In the World of Regular Expressions 121
What Are Regular Expressions? 121
Using Simple Patterns 122
Unicode Properties 123
About Metacharacters 123
Simple Quantifiers 124
Grouping in Patterns 125
Alternatives 127
Character Classes 128
Character Class Shortcuts 129
Negating the Shortcuts 131
Exercises 131
8.Matching with Regular Expressions 133
Matches with m// 133
Match Modifiers 134
Case-Insensitive Matching with/i 134
Matching Any Character with/s 134
Adding Whitespace with/x 135
Combining Option Modifiers 135
Choosing a Character Interpretation 136
Other Options 138
Anchors 138
Word Anchors 140
The Binding Operator=~ 141
Interpolating into Patterns 142
The Match Variables 143
The Persistence of Captures 144
Noncapturing Parentheses 145
Named Captures 146
The Automatic Match Variables 147
General Quantifiers 149
Precedence 150
Examples of Precedence 151
And There's More 152
A Pattern Test Program 152
Exercises 153
9.Processing Text with Regular Expressions 155
Substitutions with s/// 155
Global Replacements with/g 156
Different Delimiters 157
Substitution Modifiers 157
The Binding Operator 157
Nondestructive Substitutions 157
Case Shifting 158
The split Operator 159
The join Function 160
m//in List Context 161
More Powerful Regular Expressions 161
Nongreedy Quantifiers 162
Matching Multiple-Line Text 164
Updating Many Files 164
In-Place Editing from the Command Line 166
Exercises 168
10.More Control Structures 169
The unless Control Structure 169
The else Clause with unless 170
The until Control Structure 170
Expression Modifiers 171
The Naked Block Control Structure 172
The elsif Clause 173
Autoincrement and Autodecrement 174
The Value of Autoincrement 175
The for Control Structure 176
The Secret Connection Between foreach and for 178
Loop Controls 178
The last Operator 179
The next Operator 179
The redo Operator 181
Labeled Blocks 182
The Conditional Operator?: 182
Logical Operators 184
The Value of a Short Circuit Operator 184
The defined-or Operator 185
Control Structures Using Partial-Evaluation Operators 186
Exercises 188
11.Perl Modules 189
Finding Modules 189
Installing Modules 190
Using Your Own Directories 191
Using Simple Modules 193
The File::Basename Module 194
Using Only Some Functions from a Module 195
The File::Spec Module 196
Path::Class 197
CGI.pm 198
Databases and DBI 199
Dates and Times 200
Exercises 201
12.File Tests 203
File Test Operators 203
Testing Several Attributes of the Same File 207
Stacked File Test Operators 208
The stat and lstat Functions 210
The localtime Function 211
Bitwise Operators 212
Using Bitstrings 213
Exercises 214
13.Directory Operations 215
Moving Around the Directory Tree 215
Globbing 216
An Alternate Syntax for Globbing 217
Directory Handles 218
Recursive Directory Listing 220
Manipulating Files and Directories 221
Removing Files 221
Renaming Files 223
Links and Files 224
Making and Removing Directories 229
Modifying Permissions 230
Changing Ownership 231
Changing Timestamps 231
Exercises 232
14.Strings and Sorting 235
Finding a Substring with index 235
Manipulating a Substring with substr 236
Formatting Data with sprintf 238
Using sprintf with"Money Numbers" 238
Interpreting Non-Decimal Numerals 240
Advanced Sorting 240
Sorting a Hash by Value 244
Sorting by Multiple Keys 245
Exercises 246
15.Smart Matching and given-when 247
The Smart MaTch Operator 247
Smart Match Precedence 250
The given Statement 251
Dumb Matching 254
Using when with Many Items 256
Exercises 257
16.Process Management 259
The system Function 259
Avoiding the Shell 261
The Environment Variables 263
The exec Function 263
Using Backquotes to Capture Output 264
Using Backquotes in a List Context 267
External Processes with IPC::System::Simple 268
Processes as Filehandles 269
Getting Down and Dirty with Fork 271
Sending and Receiving Signals 272
Exercises 274
17.Some Advanced Perl Techniques 277
Slices 277
Array Slice 279
Hash Slice 281
Trapping Errors 282
Using eval 282
More Advanced Error Handling 286
autodie 288
Picking Items from a List with grep 289
Transforming Items from a List with map 290
Fancier List Utilities 291
Exercises 293
A.Exercise Answers 295
B.Beyond the Llama 331
C.A Unicode Primer 343
Index 353
- 《HTML5从入门到精通 第3版》(中国)明日科技 2019
- 《少儿电子琴入门教程 双色图解版》灌木文化 2019
- 《区块链DAPP开发入门、代码实现、场景应用》李万胜著 2019
- 《Python3从入门到实战》董洪伟 2019
- 《程序逻辑及C语言编程》卢卫中,杨丽芳主编 2019
- 《幼儿园课程资源丛书 幼儿园语言教育资源》周兢编 2015
- 《高等学校“十三五”规划教材 C语言程序设计》翟玉峰责任编辑;(中国)李聪,曾志华,江伟 2019
- 《音乐语言的根基》张艺编著 2019
- 《小提琴入门新教程 第3册》王中男著 2018
- 《小提琴入门新教程 第2册》王中男编著 2017