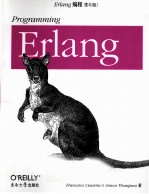
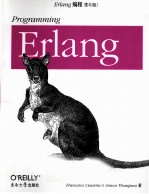
Erlang编程 英文版PDF电子书下载
- 电子书积分:15 积分如何计算积分?
- 作 者:(瑞典)塞萨芮利,(英)汤普森著
- 出 版 社:南京:东南大学出版社
- 出版年份:2010
- ISBN:9787564122690
- 页数:474 页
1.Introduction 1
Why Should I Use Erlang? 1
The History of Erlang 3
Erlang's Characteristics 4
High-Level Constructs 4
Concurrent Processes and Message Passing 5
Scalable,Safe,and Efficient Concurrency 6
Soft Real-Time Properties 6
Robustness 6
Distributed Computation 7
Integration and Openness 8
Erlang and Multicore 9
Case Studies 10
The AXD301 ATM Switch 10
CouchDB 11
Comparing Erlang to C++ 12
How Should I Use Erlang? 14
2.Basic Erlang 15
Integers 15
The Erlang Shell 16
Floats 17
Mathematical Operators 17
Atoms 19
Booleans 20
Tuples 21
Lists 22
Characters and Strings 22
Atoms and Strings 23
Building and Processing Lists 24
List Functions and Operations 25
Term Comparison 28
Variables 30
Complex Data Structures 32
Pattern Matching 33
Functions 38
Modules 40
Compilation and the Erlang Virtual Machine 40
Module Directives 41
Exercises 43
3.Sequential Erlang 45
Conditional Evaluations 46
The case Construct 46
Variable Scope 48
The if Construct 49
Guards 50
Built-in Functions 53
Object Access and Examination 53
Type Conversion 54
Process Dictionary 55
Meta Programming 55
Process,Port,Distribution,and System Information 56
Input and Output 57
Recursion 59
Tail-Recursive Functions 63
Tail-Call Recursion Optimization 66
Iterations Versus Recursive Functions 67
Runtime Errors 68
Handling Errors 70
Using try... catch 70
Using catch 74
Library Modules 77
Documentation 77
Useful Modules 79
The Debugger 80
Exercises 82
4.Concurrent Programming 89
Creating Processes 90
Message Passing 92
Receiving Messages 94
Selective and Nonselective Receives 97
An Echo Example 100
Registered Processes 102
Timeouts 104
Benchmarking 106
Process Skeletons 107
Tail Recursion and Memory Leaks 108
A Case Study on Concurrency-Oriented Programming 110
Race Conditions,Deadlocks,and Process Starvation 112
The Process Manager 114
Exercises 115
5.Process Design Patterns 117
Client/Server Models 118
A Client/Server Example 119
A Process Pattern Example 125
Finite State Machines 126
An FSM Example 127
A Mutex Semaphore 129
Event Managers and Handlers 131
A Generic Event Manager Example 132
Event Handlers 135
Exercises 137
6.Process Error Handling 139
Process Links and Exit Signals 139
Trapping Exits 142
The monitor BIFs 144
The exit BIFs 145
BIFs and Terminology 146
Propagation Semantics 148
Robust Systems 148
Monitoring Clients 150
A Supervisor Example 152
Exercises 154
7.Recordsand Macros 157
Records 158
Introducing Records 158
Working with Records 159
Functions and Pattern Matching over Records 160
Records in the Shell 161
Record Implementation 162
Record BIFs 164
Macros 165
Simple Macros 165
Parameterized Macros 166
Debugging and Macros 166
Include Files 168
Exercises 168
8.Software Upgrade 173
Upgrading Modules 173
Behind the Scenes 176
Loading Code 179
The Code Server 180
Purging Modules 182
Upgrading Processes 182
The .erlang File 186
Exercise 186
9.More Data Types and High-Level Constructs 189
Functional Programming for Real 189
Funs and Higher-Order Functions 190
Functions As Arguments 190
Writing Down Functions:fun Expressions 192
Functions As Results 193
Using Already Defined Functions 194
Functions and Variables 195
Predefined,Higher-Order Functions 195
Lazy Evaluation and Lists 197
List Comprehensions 198
A First Example 198
General List Comprehensions 198
Multiple Generators 200
Standard Functions 200
Binaries and Serialization 201
Binaries 202
The Bit Syntax 203
Pattern-Matching Bits 205
Bitstring Comprehensions 206
Bit Syntax Example:Decoding TCP Segments 206
Bitwise Operators 208
Serialization 208
References 210
Exercises 211
10.ETSand DetsTables 213
ETS Tables 213
Implementations and Trade-offs 214
Creating Tables 216
Handling Table Elements 217
Example:Building an Index,Act Ⅰ 218
Traversing Tables 220
Example:Building an Index,Act Ⅱ 222
Extracting Table Information:match 223
Extracting Table Information:select 225
Other Operations on Tables 226
Records and ETS Tables 226
Visualizing Tables 228
Dets Tables 229
A Mobile Subscriber Database Example 231
The Database Backend Operations 232
The Database Server 237
Exercises 242
11.Distributed Programming in Erlang 245
Distributed Systems in Erlang 245
Distributed Computing in Erlang:The Basics 247
Node Names and Visibility 249
Communication and Security 250
Communication and Messages 252
Node Connections 253
Remote Procedure Calls 256
The rpc Module 258
Essential Distributed Programming Modules 258
The epmd Process 260
Distributed Erlang Behind Firewalls 261
Exercises 261
12.OTP Behaviors 263
Introduction to OTP Behaviors 263
Generic Servers 266
Starting Your Server 266
Passing Messages 268
Stopping the Server 270
The Example in Full 271
Running gen_server 273
Supervisors 276
Supervisor Specifications 277
Child Specifications 278
Supervisor Example 279
Dynamic Children 280
Applications 281
Directory Structure 282
The Application Resource File 283
Starting and Stopping Applications 284
The Application Monitor 287
Release Handling 287
Other Behaviors and Further Reading 290
Exercises 291
13.Introducing Mnesia 293
When to Use Mnesia 293
Configuring Mnesia 295
Setting Up the Schema 295
Starting Mnesia 296
Mnesia Tables 296
Transactions 299
Writing 299
Reading and Deleting 300
Indexing 301
Dirty Operations 302
Partitioned Networks 304
Further Reading 305
Exercises 306
14.GUI Programming with wxErlang 309
wxWidgets 309
wxErlang:An Erlang Binding for wxWidgets 310
Objects and Types 311
Event Handling,Object Identifiers,and Event Types 312
Putting It All Together 313
A First Example:MicroBlog 314
The MiniBlog Example 317
Obtaining and Running wxErlang 321
Exercises 321
15.Socket Programming 323
User Datagram Protocol 323
Transmission Control Protocol 327
A TCP Example 328
The inet Module 331
Further Reading 333
Exercises 334
16.Interfacing Erlang with Other Programming Languages 335
An Overview of Interworking 336
Interworking with Java 337
Nodes and Mailboxes 337
Representing Erlang Types 338
Communication 338
Putting It Together:RPC Revisited 339
Interaction 340
The Small Print 341
Taking It Further 342
C Nodes 342
Going Further 345
Erlang from the Unix Shell:erl_call 346
Port Programs 346
Erlang Port Commands 347
Communicating Data to and from a Port 349
Library Support for Communication 350
Working in Ruby:erlectricity 351
Linked-in Drivers and the FFI 352
Exercises 353
17.Trace BIFs,the dbg Tracer,and Match Specifications 355
Introduction 355
The Trace BIFs 357
Process Trace Flags 358
Inheritance Flags 360
Garbage Collection and Timestamps 361
Tracing Calls with the trace_pattern BIF 362
The dbg Tracer 365
Getting Started with dbg 366
Tracing and Profiling Functions 369
Tracing Local and Global Function Calls 369
Distributed Environments 371
Redirecting the Output 371
Match Specifications:The fun Syntax 374
Generating Specifications Using fun2ms 375
Difference Between ets and dbg Match Specifications 382
Match Specifications:The Nuts and Bolts 383
The Head 383
Conditions 384
The Specification Body 387
Saving Match Specifications 390
Further Reading 391
Exercises 392
18.Types and Documentation 395
Types in Erlang 395
An Example:Records with Typed Fields 395
Erlang Type Notation 396
TypEr:Success Types and Type Inference 399
Dialyzer:A DIscrepancy AnaLYZer for ERlang Programs 401
Documentation with EDoc 402
Documenting usr_db.erl 403
Running EDoc 405
Types in EDoc 407
Going Further with EDoc 408
Exercises 410
19.EUnit and Test-Driven Development 411
Test-Driven Development 411
EUnit 412
How to Use EUnit 413
Functional Testing,an Example:Tree Serialization 413
The EUnit Infrastructure 416
Assert Macros 416
Test-Generating Functions 416
EUnit Test Representation 417
Testing State-Based Systems 418
Fixtures:Setup and Cleanup 418
Testing Concurrent Programs in Erlang 419
Exercises 420
20.Style and Efficiency 421
Applications and Modules 421
Libraries 422
Dirty Code 423
Interfaces 423
Return Values 424
Internal Data Structures 425
Processes and Concurrency 426
Stylistic Conventions 430
Coding Strategies 435
Efficiency 437
Sequential Programming 437
Lists 439
Tail Recursion and Non-tail Recursion 440
Concurrency 440
And Finally... 442
Appendix:Using Erlang 445
Index 451
- 《卓有成效的管理者 中英文双语版》(美)彼得·德鲁克许是祥译;那国毅审校 2019
- 《程序逻辑及C语言编程》卢卫中,杨丽芳主编 2019
- 《AutoCAD 2018自学视频教程 标准版 中文版》CAD/CAM/CAE技术联盟 2019
- 《跟孩子一起看图学英文》张紫颖著 2019
- 《AutoCAD机械设计实例精解 2019中文版》北京兆迪科技有限公司编著 2019
- 《全国职业院校工业机器人技术专业规划教材 工业机器人现场编程》(中国)项万明 2019
- 《复分析 英文版》(中国)李娜,马立新 2019
- 《编程超有趣 奇妙Python轻松学 第1辑》HelloCode人工智能国际研究组 2018
- 《张世祥小提琴启蒙教程 中英文双语版》张世祥编著 2017
- 《我的第一套编程启蒙绘本 看事件 开始了》编程猫教研团队编绘 2019
- 《中风偏瘫 脑萎缩 痴呆 最新治疗原则与方法》孙作东著 2004
- 《水面舰艇编队作战运筹分析》谭安胜著 2009
- 《王蒙文集 新版 35 评点《红楼梦》 上》王蒙著 2020
- 《TED说话的力量 世界优秀演讲者的口才秘诀》(坦桑)阿卡什·P.卡里亚著 2019
- 《燕堂夜话》蒋忠和著 2019
- 《经久》静水边著 2019
- 《魔法销售台词》(美)埃尔默·惠勒著 2019
- 《微表情密码》(波)卡西亚·韦佐夫斯基,(波)帕特里克·韦佐夫斯基著 2019
- 《看书琐记与作文秘诀》鲁迅著 2019
- 《酒国》莫言著 2019