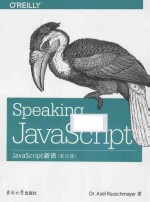
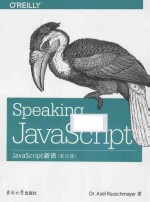
JavaScript新语 英文PDF电子书下载
- 电子书积分:14 积分如何计算积分?
- 作 者:(德)劳施迈耶著
- 出 版 社:南京:东南大学出版社
- 出版年份:2015
- ISBN:9787564153892
- 页数:440 页
Part Ⅰ.JavaScript Quick Start 3
1.Basic JavaScript 3
Background 3
Syntax 4
Variables and Assignment 6
Values 7
Booleans 12
Numbers 14
Operators 15
Strings 15
Statements 16
Functions 18
Exception Handling 21
Strict Mode 21
Variable Scoping and Closures 22
Objects and Constructors 24
Arrays 28
Regular Expressions 31
Math 31
Other Functionality of the Standard Library 32
Part Ⅱ.Background 35
2.Why JavaScript? 35
Is JavaScript Freely Available? 35
Is JavaScript Elegant? 35
Is JavaScript Useful? 36
Does JavaScript Have Good Tools? 37
Is JavaScript Fast Enough? 37
Is JavaScript Widely Used? 38
Does JavaScript Have a Future? 38
Conclusion 38
3.The Nature of JavaScript 39
Quirks and Unorthodox Features 40
Elegant Parts 40
Influences 41
4.How JavaScript Was Created 43
5.Standardization:ECMAScript 45
6.Historical JavaScript Milestones 47
Part Ⅲ.JavaScript in Depth 53
7.JavaScript's Syntax 53
An Overview of the Syntax 53
Comments 54
Expressions Versus Statements 54
Control Flow Statements and Blocks 57
Rules for Using Semicolons 57
Legal Identifiers 60
Invoking Methods on Number Literals 62
Strict Mode 62
8.Values 67
JavaScript's Type System 67
Primitive Values Versus Objects 69
Primitive Values 69
Objects 70
undefined and null 71
Wrapper Objects for Primitives 75
Type Coercion 77
9.Operators 81
Operators and Objects 81
Assignment Operators 81
Equality Operators:===Versus== 83
Ordering Operators 87
The Plus Operator(+) 88
Operators for Booleans and Numbers 89
Special Operators 89
Categorizing Values via typeof and instanceof 92
Object Operators 95
10.Booleans 97
Converting to Boolean 97
Logical Operators 99
Equality Operators,Ordering Operators 102
The Function Boolean 102
11.Numbers 103
Number Literals 103
Converting to Number 104
Special Number Values 106
The Internal Representation of Numbers 111
Handling Rounding Errors 112
Integers in JavaScript 114
Converting to Integer 117
Arithmetic Operators 122
Bitwise Operators 124
The Function Number 127
Number Constructor Properties 128
Number Prototype Methods 128
Functions for Numbers 131
Sources for This Chapter 132
12.Strings 133
String Literals 133
Escaping in String Literals 134
Character Access 135
Converting to String 135
Comparing Strings 136
Concatenating Strings 137
The Function String 138
String Constructor Method 138
String Instance Property length 139
String Prototype Methods 139
13.Statements 145
Declaring and Assigning Variables 145
The Bodies of Loops and Conditionals 145
Loops 146
Conditionals 150
The with Statement 153
The debugger Statement 155
14.ExceptionHandling 157
What Is Exception Handling? 157
Exception Handling in JavaScript 158
Error Constructors 161
Stack Traces 162
Implementing Your Own Error Constructor 163
15.Functions 165
The Three Roles of Functions in JavaScript 165
Terminology:"Parameter"Versus"Argument" 166
Defining Functions 166
Hoisting 168
The Name of a Function 169
Which Is Better:A Function Declaration or a Function Expression? 169
More Control over Function Calls:call(),apply(),and bind() 170
Handling Missing or Extra Parameters 171
Named Parameters 176
16.Variables:Scopes,Environments,and Closures 179
Declaring a Variable 179
Background:Static Versus Dynamic 179
Background:The Scope of a Variable 180
Variables Are Function-Scoped 181
Variable Declarations Are Hoisted 182
Introducing a New Scope via an IIFE 183
Global Variables 186
The Global Object 188
Environments:Managing Variables 190
Closures:Functions Stay Connected to Their Birth Scopes 193
17.Objects and Inheritance 197
Layer 1:Single Objects 197
Converting Any Value to an Object 203
this as an Implicit Parameter of Functions and Methods 204
Layer 2:The Prototype Relationship Between Objects 211
Iteration and Detection of Properties 217
Best Practices:Iterating over Own Properties 220
Accessors(Getters and Setters) 221
Property Attributes and Property Descriptors 222
Protecting Objects 229
Layer 3:Constructors—Factories for Instances 231
Data in Prototype Properties 241
Keeping Data Private 244
Layer 4:Inheritance Between Constructors 251
Methods of All Objects 257
Generic Methods:Borrowing Methods from Prototypes 260
Pitfalls:Using an Object as a Map 266
Cheat Sheet:Working with Objects 270
18.Arrays 273
Overview 273
Creating Arrays 274
Array Indices 276
length 279
Holes in Arrays 282
Array Constructor Method 285
Array Prototype Methods 286
Adding and Removing Elements(Destructive) 286
Sorting and Reversing Elements(Destructive) 287
Concatenating,Slicing,Joining(Nondestructive) 289
Searching for Values(Nondestructive) 290
Iteration (Nondestructive) 291
Pitfall:Array-Like Objects 295
Best Practices:Iterating over Arrays 295
19.Regular Expressions 297
Regular Expression Syntax 297
Unicode and Regular Expressions 302
Creating a Regular Expression 302
RegExp.prototype.test:Is There a Match? 304
String.prototype.search:At What Index Is There a Match? 305
RegExp.prototype.exec:Capture Groups 305
String.prototype.match:Capture Groups or Return All Matching Substrings 307
String.prototype.replace:Search and Replace 307
Problems with the Flag/g 309
Tips andTricks 311
Regular Expression Cheat Sheet 314
20.Dates 317
The Date Constructor 317
Date Constructor Methods 318
Date Prototype Methods 319
Date Time Formats 322
Time Values:Dates as Milliseconds Since 1970-01-01 324
21.Math 327
Math Properties 327
Numerical Functions 328
Trigonometric Functions 329
Other Functions 330
22.JSON 333
Background 333
JSON.stringify(value,replacer?,space?) 337
JSON.parse(text,reviver?) 340
Transforming Data via Node Visitors 341
23.StandardGlobalVariables 345
Constructors 345
Error Constructors 345
Nonconstructor Functions 346
Dynamically Evaluating JavaScript Code via eval()and new Function() 347
The Console API 351
Namespaces and Special Values 356
24.Unicodeand JavaScript 357
Unicode History 357
Important Unicode Concepts 357
Code Points 359
Unicode Encodings 359
JavaScript Source Code and Unicode 361
JavaScript Strings and Unicode 364
JavaScript Regular Expressions and Unicode 365
25.Newin ECMAScript5 369
New Features 369
Syntactic Changes 370
New Functionality in the Standard Library 370
Tips for Working with Legacy Browsers 372
Part Ⅳ.Tips,Tools,and Libraries 375
26.A Meta Code Style Guide 375
Existing Style Guides 375
General Tips 375
Commonly Accepted Best Practices 377
Controversial Rules 382
Conclusion 386
27.Language Mechanisms for Debugging 387
28.Subclassing Built-ins 389
Terminology 389
Obstacle 1:Instances with Internal Properties 389
Obstacle 2:A Constructor That Can't Be Called as a Function 392
Another Solution:Delegation 393
29.JSDoc:Generating API Documentation 395
The Basics of JSDoc 396
BasicTags 397
Documenting Functions and Methods 399
Inline Type Information ("Inline Doc Comments") 399
Documenting Variables,Parameters,and Instance Properties 400
Documenting Classes 401
Other Useful Tags 403
30.Libraries 405
Shims Versus Polyfills 405
Four Language Libraries 406
The ECMAScript Internationalization API 406
Directories for JavaScript Resources 408
31.Module Systems and Package Managers 411
Module Systems 411
Package Managers 412
Quick and Dirty Modules 412
32.MoreTools 415
33.WhattoDoNext 417
Index 419
- 《世说新语校笺 第1册》(南朝宋)刘义庆撰;(南朝梁)刘孝标注;杨勇校笺 2019
- 《世说新语今注今译》(南朝宋)刘义庆编撰;陈引驰注译 2018
- 《戴建业精读世说新语》汪超毅责任编辑;戴建业 2019
- 《且说新语》王志清编著 2014
- 《岁岁新语系列 鲜段大汇 2012》辛言编著 2012
- 《小小说新语》王彦艳主编 2014
- 《群书治要译注 第23册 卷39 吕氏春秋 卷40 韩子三略 新语贾子》(唐)魏徵,褚亮,虞世南,萧德言编;《群书治要》学习小组译注 2012
- 《蒋星煜文集 第1卷 中国隐士与中国文化·颜鲁公之书学·史林新语》蒋星煜著 2013
- 《高职新语文》吴梅菊主编 2012
- 《周有光文集 第5卷 新语文的建设 新时代的新语文》周有光著 2013
- 《中风偏瘫 脑萎缩 痴呆 最新治疗原则与方法》孙作东著 2004
- 《水面舰艇编队作战运筹分析》谭安胜著 2009
- 《王蒙文集 新版 35 评点《红楼梦》 上》王蒙著 2020
- 《TED说话的力量 世界优秀演讲者的口才秘诀》(坦桑)阿卡什·P.卡里亚著 2019
- 《燕堂夜话》蒋忠和著 2019
- 《经久》静水边著 2019
- 《魔法销售台词》(美)埃尔默·惠勒著 2019
- 《微表情密码》(波)卡西亚·韦佐夫斯基,(波)帕特里克·韦佐夫斯基著 2019
- 《看书琐记与作文秘诀》鲁迅著 2019
- 《酒国》莫言著 2019