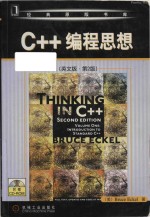
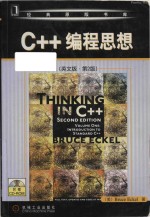
Thinking in C++ Volume 2: Practical Programming = C++编程思想 第2卷 实用编程技术 (英文版)PDF电子书下载
- 电子书积分:22 积分如何计算积分?
- 作 者:Bruce Eckel ; Chuck Allison
- 出 版 社:China Machine Press
- 出版年份:2002
- ISBN:7111121880
- 页数:802 页
Preface 1
What’s new in the second edition 2
What’s in Volume 2 of this book 3
How to get Volume 2 3
Prerequisites 3
Learning C++ 4
Goals 5
Chapters 7
Exercises 12
Exercise solutions 12
Source code 12
Language standards 14
Language support 15
The book’s CD ROM 15
CD ROMs,seminars,and consulting 16
Errors 16
About the cover 17
Book design and production 18
Acknowledgements 19
1:Introduction to Objects 21
The progress of abstraction 22
An object has an interface 25
The hidden implementation 28
Reusing the implementation 29
Inheritance:reusing the interface 31
Is-a vs.is-like-a relationships 35
Interchangeable objects with polymorphism 37
Creating and destroying objects 41
Exception handling:dealing with errors 43
Analysis and design 44
Phase 0:Make a plan 47
Phase 1:What a we making? 48
Phase 2:How will we build it? 52
Phase 3:Build the core 56
Phase 4:Iterate the use cases 57
Phase 5:Evolution 58
Plans pay off 60
Extreme programming 61
Write tests first 61
Pair programming 63
Why C++ succeeds 64
A better C 65
You’re already on the learning curve 65
Efficiency 66
Systems are easier to express and understand 66
Maximal leverage with libraries 67
Source-code reuse with templates 67
Error handling 67
Programming in the large 68
Strategies for transition 68
Guidelines 69
Management obstacles 71
Summary 73
2:Making & Using Objects 75
The process of language translation 76
Interpreters 77
Compilers 77
The compilation process 79
Tools for separate compilation 80
Declarations vs.definitions 81
Linking 87
Using libraries 88
Your first C++ program 90
Using the lostreams class 90
Namespaces 91
Fundamentals of program structure 93
“Hello,world!” 94
Running the compiler 95
More about iostreams 96
Character array concatenation 96
Reading input 97
Calling other programs 98
Introducing strings 98
Reading and writing files 100
Introducing vector 102
Summary 108
Exercises 109
3:The C in C++ 111
Creating functions 112
Function return values 115
Using the C function library 116
Creating your own braries with the librarian 117
Controlling execution 117
True and false 117
if-else 118
while 119
do-while 120
for 121
The break and continue keywords 122
switch 123
Using and misusing goto 125
Recursion 126
Introduction to operators 127
Precedence 127
Auto increment and decrement 128
Introduction to data types 129
Basic built-in types 129
bool,true,& false 131
Specifiers 132
Introduction to pointers 133
Modifying the outside object 137
Introduction to C++ references 140
Pointers and references as modifiers 141
Scoping 143
Defining variables on the fly 145
Specifying storage allocation 147
Global variables 147
Local variables 149
static 149
extem 151
Constants 153
volatile 155
Operators and their use 156
Assignment 156
Mathematical operators 156
Relational operators 158
Logical operators 158
Bitwise operators 159
Shift operators 160
Unary operators 163
The ternary operator 164
The comma operator 165
Common pitfalls when using operators 166
Casting operators 166
C++ explicit casts 167
sizeof - an operator by itself 172
The asm keyword 173
Explicit operators 173
Composite type creation 174
Aliasing names with typedef 174
Combining variables with struct 175
Clarifying programs with enum 179
Saving memory with union 181
Arrays 182
Debugging hints 193
Debugging flags 194
Turning variables and expressions into strings 196
The C assert( ) macro 197
Function addresses 198
Defining a function pointer 198
Complicated declarations & definitions 199
Using a function pointer 200
Arrays of pointers to functions 201
Make:managing separate compilation 202
Make activities 204
Makefiles in this book 207
An example makefile 208
Summary 210
Exercises 210
4:Data Abstraction 217
A tiny C-like library 219
Dynamic storage allocation 223
Bad guesses 227
What’s wrong? 229
The basic object 230
What’s an object? 238
Abstract data typing 239
Object details 240
Header file etiquette 241
Importance of header files 242
The multiple-declaration problem 244
The preprocessor directives #define,#ifdef,and #endif 245
A standard for header files 246
Namespaces in headers 247
Using headers in projects 248
Nested structures 248
Global scope resolution 253
Summary 253
Exercises 254
5:Hiding the Implementation 259
Setting limits 260
C++ access control 261
protected 263
Friends 263
Nested friends 266
Is it pure? 269
Object layout 269
The class 270
Modifying Stash to use access control 273
Modifying Stack to use access control 274
Handle classes 275
Hiding the implementation 276
Reducing recompilation 276
Summary 279
Exercises 280
6:Initialization &Cleanup 283
Guaranteed initialization with the constructor 285
Guaranteed cleanup with the destructor 287
Elimination of the definition block 289
for loops 291
Storage allocation 292
Stash with constructors and destructors 294
Stack with constructors & destructors 298
Aggregate initialization 301
Default constructors 304
Summary 305
Exercises 306
7:Function Overloading & Default Arguments 309
More name decoration 311
Overloading on return values 312
Type-safe linkage 313
Overloading example 314
unions 318
Default arguments 321
Placeholder arguments 323
Choosing overloading vs.default arguments 324
Summary 329
Exercises 330
8:Constants 333
Value substitution 334
const in header files 335
Safety consts 336
Aggregates 337
Differences with C 338
Pointers 340
Pointer to const 340
const pointer 341
Assignment and pe checking 343
Function arguments & return values 344
Passing by const value 344
Returning by const value 345
passing and returning addresses 349
Classes 352
const in classes 353
Compile-time constants in classes 356
const objects &member functions 359
volatile 365
Summary 367
Exercises 367
9:Inline Functions 371
Preprocessor pitfalls 372
Macros and access 376
Inline functions 377
Inlines inside classes 378
Access functions 379
Stash & Stack with inlines 385
Inlines &the compiler 390
Limitations 390
Forward references 391
Hidden activities in constructors &destructors 392
Reducing clutter 393
More preprocessor features 395
Token pasting 396
Improved error checking 396
Summary 400
Exercises 400
10:Name Control 405
Static elements from C 406
static variables inside functions 406
Controlling linkage 412
Other storage class specifiers 414
Namespaces 414
Creating a namespace 415
Using a namespace 417
The use of namespaces 422
Static members in C++ 423
Defining storage for static data members 424
Nested and local classes 428
static member functions 429
Static initialization dependency 432
What to do 434
Alternate linkage specifications 442
Summary 443
Exercises 443
11:References & the Copy-Constructor 449
Pointers in C++ 450
References in C++ 451
References in functions 452
Argument-passing guidelines 455
The copy-constructor 455
Passing & returning by value 455
Copy-construction 462
Default copy-constructor 468
Altematives to copy-construction 471
Pointers to members 473
Functions 475
Summary 478
Exercises 479
12:Operator Overloading 485
Warning &reassurance 486
Syntax 487
Overloadable operators 488
Unary operators 489
Binary operators 493
Arguments &return values 505
Unusual operators 508
Operators you can’t overload 517
Non-member operators 518
Basic guidelines 520
Overloading assignment 521
Behavior of operator= 522
Automatic type conversion 533
Constructor conversion 534
Operator conversion 535
Type conversion example 538
Pitfalls in automatic type conversion 539
Summary 542
Exercises 542
13:Dynamic Object Creation 547
Object creation 549
C’s approach to the heap 550
operator new 552
operator delete 553
A simple example 553
Memory manager overhead 554
Early examples redesigned 555
delete void* is probably a bug 555
Cleanup responsibility with pointers 557
Stash for pointers 558
new & delete for arrays 563
Making a pointer more like an array 564
Running out of storage 565
Overloading new & delete 566
Overloading global new & delete 568
Overloading new & delete for a class 570
Overloading new & delete for arrays 573
Constructor calls 576
placement new & delete 577
Summary 580
Exercises 580
14:Inheritance & Composition 583
Composition syntax 584
Inheritance syntax 586
The constructor initializer list 588
Member object initialization 589
Built-in types in the initializer list 589
Combining composition & inheritance 591
Order of constructor &destructor calls 592
Name hiding 595
Functions that don’t automatically inherit 600
Inheritance and static member functions 604
Choosing composition vs.inheritance 604
Subtyping 606
private inheritance 609
protected 610
protected inheritance 611
Operator overloading & inheritance 612
Multiple inheritance 613
Incremental development 614
Upcasting 615
Why “upcasting?” 617
Upcasting and the copy-constructor 617
Composition vs.inheritance (revisited) 620
Pointer & reference upcasting 622
A crisis 622
Summary 623
Exercises 623
15:Polymorphism &Virtual Functions 627
Evolution of C++programmers 628
Upcasting 629
The problem 631
Function call binding 631
virtual functions 632
Extensibility 633
How C++ implements late binding 636
Storing type information 637
Picturing virtual functions 639
Under the hood 642
Installing the vpointer 643
Objects are different 644
Why virtual functions? 645
Abstract base classes and pure virtual functions 646
Pure virtual definitions 651
Inheritance and the VTABLE 652
Object slicing 655
Overloading &overriding 658
Variant return type 660
virtual functions &constructors 662
Order of constructor calls 663
Behavior of virtual functions inside constructors 664
Destructors and virtual destructors 665
Pure virtual destructors 668
Virtuals in destructors 670
Creating an object-based hierarchy 671
Operator overloading 675
Downcasting 678
Summary 681
Exercises 682
16:Introduction to Templates 689
Containers 690
The need for containers 692
Overview of templates 693
The template solution 696
Template syntax 697
Non-inline function definitions 699
IntStack as a template 701
Constants in templates 703
Stack and Stash as templates 705
Templatized pointer Stash 707
Turning ownership on and off 713
Holding objects by value 716
Introducing iterators 719
Stack with iterators 728
PStash with iterators 732
Why iterators? 738
Function templates 742
Summary 743
Exercises 744
A:Coding Style 747
B:Programming Guidelines 759
C:Recommended Reading 775
C 776
General C++ 776
My own list of tooks 777
Depth &dark corners 778
Analysis & design 779
Index 783
- 《钒产业技术及应用》高峰,彭清静,华骏主编 2019
- 《现代水泥技术发展与应用论文集》天津水泥工业设计研究院有限公司编 2019
- 《异质性条件下技术创新最优市场结构研究 以中国高技术产业为例》千慧雄 2019
- 《Prometheus技术秘笈》百里燊 2019
- 《中央财政支持提升专业服务产业发展能力项目水利工程专业课程建设成果 设施农业工程技术》赵英编 2018
- 《药剂学实验操作技术》刘芳,高森主编 2019
- 《林下养蜂技术》罗文华,黄勇,刘佳霖主编 2017
- 《脱硝运行技术1000问》朱国宇编 2019
- 《催化剂制备过程技术》韩勇责任编辑;(中国)张继光 2019
- 《信息系统安全技术管理策略 信息安全经济学视角》赵柳榕著 2020
- 《少数民族作家海外推广系列 祭语风中》Bruce Humes,范伟责任编辑;(美)Joshua Dyer译;(中国)次仁罗布 2019
- 《量子力学=Quantum Mechanics(Jones & Bartlett):英文》(美)B.C.里德(Bruce Cameron Reed)著 2018
- 《重建 重塑婚姻与自我的愿景》布鲁斯·费雪(BRUCE FISHER)博士,罗伯·艾伯提(ROBERT E .ALBERTI)博士合著;张美惠译 2003
- 《大学英语ESP快速阅读教程 中级》贾爱武总主编;张艺宁主编;(美)CHUCK WHEELER审订;丁仁仑,郭虹宇,江丹邓编委 2012
- 《高速信令 抖动建模、分析与预算》(美)KyungSuk(Dan)Oh,(美)Xingchao(Chuck)Yuan主编;李玉山等译 2014
- 《科学学习心理学》Shawn M. Glynn,Russell H. Yeany,Bruce K. Britton著;熊召弟等译 1996
- 《泌乳》(美)B.L.拉森(Bruce L. Larson)主编;王秋芬等译 1992
- 《当代澳大利亚中短篇小说选》朱炯强,(澳)帕斯科(Pascoe,Bruce)主编 1992
- 《PowerBuilder 应用程序开发指南》(美)Kent marsh,(美)Bruce Braunstein著;廖卫东等译 1996
- 《实用林木改良》(美)佐贝尔(Zobel,Bruce J.)等著;王章荣等译 1990
- 《R语言机器学习 原书第2版=MACHINE LEARNING USING R WITH TIME SERIES AND INDUSTRY-BASED USE CASES IN R》SECOND EDITION
- 《机器学习实战 基于SOPHON平台的机器学习理论与实践=MACHINE LEARNING IN ACTION PRINCIPLES AND PRACTICE BASED ON TH》星环科技人工智能平台团队编著 2020
- 《竞争战略 全译珍藏版》(美)迈克尔·波特(Michael E. Porter)著 2012
- 《网络互联技术手册 第2版》(美)(K.唐斯)Kevin Downes等著;包晓露等译 1999
- 《新版交换式以太网和快速型以太网 第2版》(美)(R.布雷耶)Robert Breyer,(美)(S.赖利)Sean Riley著;肖文贵等译 1997
- 《摄影100关键词》(英)克拉克著 2011
- 《数控技术专业英语》高成秀主编 2010
- 《守望百年 中英文对照爱情长诗》蔡丽双著;张智中译 2014
- 《环境政策概要》(英)卡罗琳·斯奈尔(Carolyn Snell)著;宋伟译 2017
- 《驼铃 中-英-波兰文对照诗集》蔡丽双著;张智中,(波兰)博古米娜·雅尼卡译 2015